c++怎么用线程池
时间: 2023-06-29 10:17:29 浏览: 43
在C++中,可以使用`std::thread`和`std::condition_variable`等标准库组件来实现线程池。
一个线程池通常包含一个任务队列和一组工作线程。当有新任务需要执行时,线程池会将任务放入任务队列中,并唤醒一个工作线程来执行任务。当工作线程完成任务后,它会回到线程池中等待下一个任务。
下面是一个简单的线程池实现:
```c++
#include <thread>
#include <vector>
#include <queue>
#include <mutex>
#include <condition_variable>
class ThreadPool
{
public:
ThreadPool(size_t numThreads)
{
for (size_t i = 0; i < numThreads; i++)
{
threads.emplace_back([this]
{
while (true)
{
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(this->queue_mutex);
this->condition.wait(lock, [this] { return this->stop || !this->tasks.empty(); });
if (this->stop && this->tasks.empty())
return;
task = std::move(this->tasks.front());
this->tasks.pop();
}
task();
}
});
}
}
template<class F>
void enqueue(F&& f)
{
{
std::unique_lock<std::mutex> lock(queue_mutex);
tasks.emplace(std::forward<F>(f));
}
condition.notify_one();
}
~ThreadPool()
{
{
std::unique_lock<std::mutex> lock(queue_mutex);
stop = true;
}
condition.notify_all();
for (std::thread& thread : threads)
thread.join();
}
private:
std::vector<std::thread> threads;
std::queue<std::function<void()>> tasks;
std::mutex queue_mutex;
std::condition_variable condition;
bool stop = false;
};
```
在上面的代码中,我们定义了一个名为`ThreadPool`的类,该类包含一个任务队列和一组工作线程。在类的构造函数中,我们创建了一定数量的工作线程,并将它们置于一个无限循环中,等待任务的到来。在任务到达时,每个工作线程从任务队列中取出一个任务并执行。
我们还定义了一个`enqueue()`函数,该函数接受一个可调用对象,并将其放入任务队列中。当任务到达时,我们使用`notify_one()`函数唤醒一个工作线程来执行任务。
最后,在类的析构函数中,我们设置`stop`标志,并使用`notify_all()`函数唤醒所有工作线程,让它们退出循环并结束线程。
使用线程池的示例如下:
```c++
#include <iostream>
#include <chrono>
int main()
{
ThreadPool pool(4); // 创建一个拥有4个工作线程的线程池
// 向线程池中添加一些任务
for (int i = 0; i < 8; i++)
{
pool.enqueue([i]
{
std::cout << "Task " << i << " started." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
std::cout << "Task " << i << " finished." << std::endl;
});
}
// 等待一段时间,让线程池中的任务执行完毕
std::this_thread::sleep_for(std::chrono::seconds(10));
return 0;
}
```
在上面的示例代码中,我们创建了一个拥有4个工作线程的线程池,并向线程池中添加了8个任务。每个任务都会打印一条开始和结束的消息,并在执行过程中休眠1秒钟。最后,我们等待10秒钟,让线程池中的任务执行完毕。
相关推荐
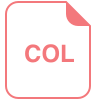
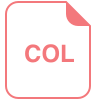
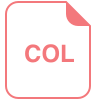
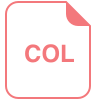
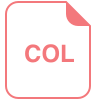










