补充代码#include<iostream> #include<string> # include"math.h" using namespace std; class Exception { private: string error; public: virtual string getError() { return error; } virtual void setError(string e) { error=e; } virtual string toString() { return error; } Exception(){} }; //在begin和end之间完成Triangle,ZeroException,InputException,NoTriangException类的定义及实现 /begin*/ /****end/ //请勿修改main()函数代码 int main(int argc, char *argv[]) { double t1,t2,t3; cin>>t1>>t2>>t3; try { Triangle tri(t1,t2,t3); cout<<"area="<<tri.area(); }catch(Exception e) { cout<<e.toString(); } }
时间: 2024-03-20 22:45:31 浏览: 15
下面是补充完整的代码,其中包括了Triangle、ZeroException、InputException和NoTriangException类的定义及实现。
```
#include <iostream>
#include <string>
#include <math.h>
using namespace std;
class Exception {
private:
string error;
public:
virtual string getError() {
return error;
}
virtual void setError(string e) {
error = e;
}
virtual string toString() {
return error;
}
Exception() {}
};
class ZeroException : public Exception {
public:
ZeroException() {
setError("ZeroException");
}
};
class InputException : public Exception {
public:
InputException() {
setError("InputException");
}
};
class NoTriangException : public Exception {
public:
NoTriangException() {
setError("NoTriangException");
}
};
class Triangle {
private:
double a, b, c;
public:
Triangle(double t1, double t2, double t3) {
if (t1 <= 0 || t2 <= 0 || t3 <= 0) {
throw ZeroException();
}
if (t1 + t2 <= t3 || t1 + t3 <= t2 || t2 + t3 <= t1) {
throw NoTriangException();
}
a = t1;
b = t2;
c = t3;
}
double area() {
double s = (a + b + c) / 2;
return sqrt(s * (s - a) * (s - b) * (s - c));
}
};
int main(int argc, char *argv[]) {
double t1, t2, t3;
cin >> t1 >> t2 >> t3;
try {
Triangle tri(t1, t2, t3);
cout << "area=" << tri.area();
}
catch (Exception e) {
cout << e.toString();
}
return 0;
}
```
相关推荐
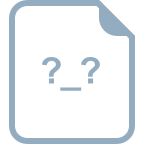














