stm32f407外部中断控制LED灯
时间: 2023-08-07 17:27:49 浏览: 204
好的,针对您的问题,我可以给您提供以下步骤:
1. 首先,需要配置外部中断,使得当外部中断触发时,能够执行相应的操作,例如控制 LED 灯的状态。
2. 然后,需要配置 LED 灯的引脚,使其能够被控制。
3. 接下来,在中断回调函数中实现对 LED 灯的控制。当外部中断触发时,中断回调函数会被调用,然后可以在其中修改 LED 灯的状态。
下面是一份示例代码,仅供参考:
```
#include "stm32f4xx.h"
#define LED_PIN GPIO_Pin_12
#define LED_PORT GPIOD
void EXTI0_IRQHandler(void)
{
if(EXTI_GetITStatus(EXTI_Line0) != RESET)
{
// 等待一段时间,以消除抖动
for(int i = 0; i < 100000; i++);
// 切换 LED 灯状态
GPIO_ToggleBits(LED_PORT, LED_PIN);
EXTI_ClearITPendingBit(EXTI_Line0);
}
}
void LED_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
// 使能 GPIOD 时钟
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOD, ENABLE);
// 配置 LED 引脚为推挽输出模式
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_PORT, &GPIO_InitStructure);
// 初始状态为关闭
GPIO_SetBits(LED_PORT, LED_PIN);
}
void EXTI_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
EXTI_InitTypeDef EXTI_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
// 使能 GPIOA 和 SYSCFG 时钟
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SYSCFG, ENABLE);
// 配置 PA0 引脚为输入模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置 EXTI0 中断线路
SYSCFG_EXTILineConfig(EXTI_PortSourceGPIOA, EXTI_PinSource0);
EXTI_InitStructure.EXTI_Line = EXTI_Line0;
EXTI_InitStructure.EXTI_Mode = EXTI_Mode_Interrupt;
EXTI_InitStructure.EXTI_Trigger = EXTI_Trigger_Falling;
EXTI_InitStructure.EXTI_LineCmd = ENABLE;
EXTI_Init(&EXTI_InitStructure);
// 配置 EXTI0 中断优先级
NVIC_InitStructure.NVIC_IRQChannel = EXTI0_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0x00;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0x00;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
}
int main(void)
{
LED_Init();
EXTI_Init();
while(1);
return 0;
}
```
这份代码实现了当 PA0 引脚触发下降沿时,切换 PD12 引脚的状态。请注意,这里的代码是针对 STM32F407VG 开发板的,如果您的硬件平台不同,需要做相应的修改。
阅读全文
相关推荐
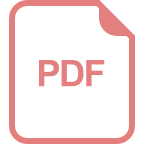

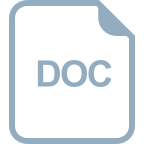
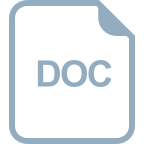





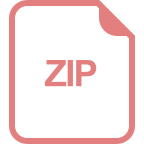
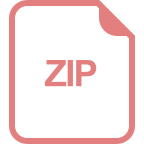





