设计一个职工文件emp.dat,包含员工工资(salary)信息,按照工资salary对所有职工记录进行递减排序。c语言完成
时间: 2024-10-23 16:18:25 浏览: 62
设计一个名为`emp.dat`的职工文件,可以使用文本文件存储每个员工的信息,例如工资(salary),通常会有一个简单的结构来表示这种数据。为了按照工资降序排列,我们可以创建一个结构体来代表员工,并使用快速排序或归并排序等算法对数据进行排序。以下是使用C语言的基本步骤:
1. 定义结构体Employee,包含工资字段:
```c
typedef struct {
int id;
double salary;
} Employee;
```
2. 打开文件进行读写操作,这里假设文件已经存在:
```c
FILE* file = fopen("emp.dat", "r+"); // 以读写模式打开
if (file == NULL) {
perror("Failed to open the file");
exit(1);
}
```
3. 定义一个函数用于比较两个员工工资并返回排序顺序:
```c
int compareSalaries(const void* a, const void* b) {
const Employee* e1 = (const Employee*)a;
const Employee* e2 = (const Employee*)b;
return (e2->salary > e1->salary ? 1 : (e2->salary < e1->salary ? -1 : 0));
}
```
4. 使用`qsort`函数对文件内容进行排序:
```c
Employee employees[100]; // 假设最多有100名员工
int numEmployees;
// 从文件中读取数据并填充数组
numEmployees = fread(employees, sizeof(Employee), sizeof(employees), file);
if (ferror(file)) {
perror("Reading from the file failed");
exit(1);
}
// 对数组进行排序
qsort(employees, numEmployees, sizeof(Employee), compareSalaries);
// 现在数组是按工资降序排列的
```
5. 排序完成后,如果需要将结果回写到文件:
```c
// 将排序后的员工信息写回到文件
rewind(file); // 重置文件指针
for (int i = 0; i < numEmployees; i++) {
fwrite(&employees[i], sizeof(Employee), 1, file);
}
if (ferror(file)) {
perror("Writing to the file failed");
exit(1);
}
fclose(file); // 关闭文件
```
阅读全文
相关推荐
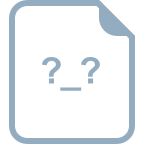
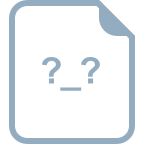
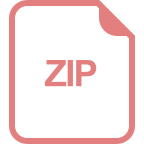















