c语言:定义一个员工结构(包含员工工号、姓名、性别),输入4个员工的数据,将这些员工数据保存到文件employer.dat中,最后按照工号从大到小顺序在屏幕上输出。
时间: 2024-03-16 21:41:45 浏览: 218
好的,以下是C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义员工结构体
struct employee {
int id; // 工号
char name[50]; // 姓名
char gender; // 性别
};
int main() {
struct employee emp[4]; // 定义4个员工
// 输入4个员工的数据
for (int i = 0; i < 4; i++) {
printf("请输入第%d个员工的工号、姓名、性别(用空格分隔):", i + 1);
scanf("%d %s %c", &emp[i].id, emp[i].name, &emp[i].gender);
}
// 将员工数据保存到文件employer.dat中
FILE *fp = fopen("employer.dat", "wb"); // 以二进制写入方式打开文件
if (fp == NULL) {
printf("文件打开失败!\n");
exit(1);
}
fwrite(emp, sizeof(struct employee), 4, fp); // 将员工信息写入文件
fclose(fp); // 关闭文件
// 按照工号从大到小顺序在屏幕上输出员工信息
fp = fopen("employer.dat", "rb"); // 以二进制读取方式打开文件
if (fp == NULL) {
printf("文件打开失败!\n");
exit(1);
}
fseek(fp, 0, SEEK_END); // 将文件指针移动到文件末尾
long size = ftell(fp); // 获取文件大小
int n = size / sizeof(struct employee); // 获取员工数量
struct employee *emp2 = (struct employee*)malloc(size); // 动态分配内存
fseek(fp, 0, SEEK_SET); // 将文件指针移动到文件开头
fread(emp2, sizeof(struct employee), n, fp); // 读取员工信息
fclose(fp); // 关闭文件
// 冒泡排序
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (emp2[j].id < emp2[j + 1].id) {
struct employee temp = emp2[j];
emp2[j] = emp2[j + 1];
emp2[j + 1] = temp;
}
}
}
// 输出排序后的员工信息
printf("按照工号从大到小顺序输出员工信息:\n");
for (int i = 0; i < n; i++) {
printf("%d %s %c\n", emp2[i].id, emp2[i].name, emp2[i].gender);
}
free(emp2); // 释放内存
return 0;
}
```
注意:在写入和读取文件时,需要以二进制方式打开文件,否则会出现乱码和数据错误。另外,为了方便排序,这里使用了冒泡排序算法。
阅读全文
相关推荐
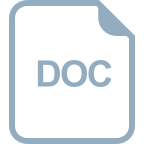
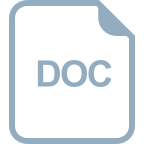
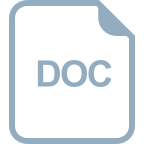

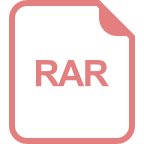
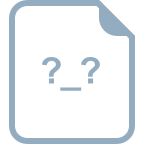
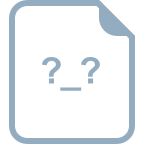
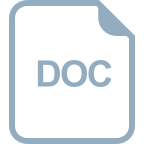
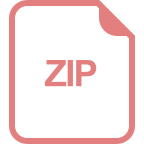
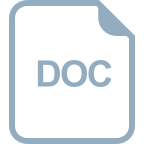
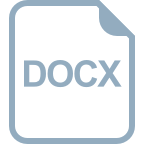
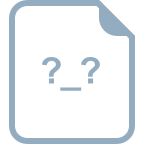
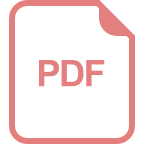
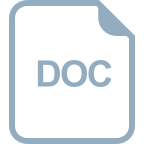
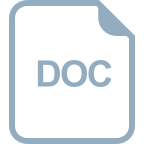
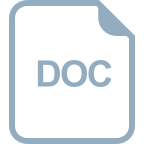
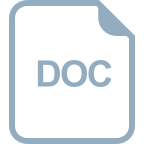

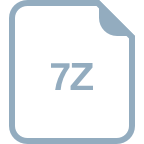