定义一方法,该方法用于一组数组的最大值。主方法中调用它。 例,可以求一个班的最高分,可以求一队伍最高个的那个人的身高。
时间: 2023-09-07 10:04:49 浏览: 64
### 回答1:
以下是 Java 语言的实现:
```java
public class MaxValue {
public static int findMax(int[] arr) {
int max = arr[0];
for (int i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
public static void main(String[] args) {
int[] scores = {85, 92, 78, 90, 88};
int maxScore = findMax(scores);
System.out.println("最高分是:" + maxScore);
int[] heights = {170, 175, 180, 165, 185};
int tallestHeight = findMax(heights);
System.out.println("最高个子的身高是:" + tallestHeight);
}
}
```
该程序定义了一个静态方法 `findMax`,接受一个整型数组作为参数,返回数组中的最大值。在主方法中,分别定义了一个成绩数组和身高数组,并分别调用 `findMax` 方法求出最高分和最高个子的身高。
### 回答2:
可以定义一个名为"findMax"的方法,该方法接收一个数组作为参数,并返回该数组中的最大值。
方法实现如下:
```
public static int findMax(int[] arr) {
int max = arr[0]; //假设数组中的第一个元素为最大值
for (int i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i]; //如果当前元素大于max,则更新max的值
}
}
return max;
}
```
在主方法中调用该方法,可以实现找到一组数组中的最大值。例如,我们可以定义一个数组来表示一个班的学生成绩:
```
public static void main(String[] args) {
int[] scores = {90, 88, 95, 78, 84};
int maxScore = findMax(scores);
System.out.println("班级最高分是:" + maxScore);
}
```
输出结果为:"班级最高分是:95"
同样,我们也可以使用这个方法来找到一队伍中身高最高的人:
```
public static void main(String[] args) {
int[] heights = {175, 180, 165, 190, 170};
int maxHeight = findMax(heights);
System.out.println("队伍中身高最高的人的身高是:" + maxHeight);
}
```
输出结果为:"队伍中身高最高的人的身高是:190"
### 回答3:
定义一个方法findMax,该方法参数为一个数组arr,返回值为数组中的最大值。
方法内部:
- 创建一个变量max,并初始化为数组第一个元素arr[0]。
- 遍历数组中的每一个元素:
- 若当前元素大于max,则更新max的值为当前元素的值。
- 遍历结束后,返回max作为最大值。
主方法中:
- 创建一个数组arr,存放需要求最大值的一组数。
- 调用findMax方法,传入arr作为参数,并将返回值存储在变量maxValue中。
- 打印maxValue的值,即为数组arr的最大值。
示例代码如下:
```java
public class Main {
// 定义方法,用于求一组数组的最大值
public static int findMax(int[] arr) {
int max = arr[0];
for (int i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
public static void main(String[] args) {
int[] scores = {85, 97, 92, 88, 90}; // 存放班级的考试成绩
int maxScore = findMax(scores); // 调用findMax方法求最高分
System.out.println("班级最高分:" + maxScore);
int[] heights = {170, 165, 180, 175, 185}; // 存放一队伍的身高
int maxHeight = findMax(heights); // 调用findMax方法求身高最高的人的身高
System.out.println("身高最高的人的身高:" + maxHeight);
}
}
```
输出结果:
```
班级最高分:97
身高最高的人的身高:185
```
相关推荐
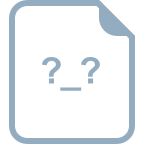
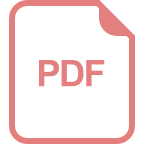
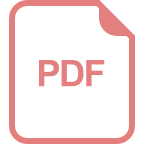
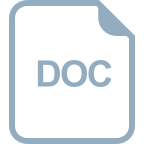
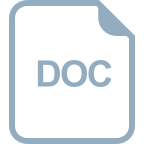
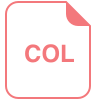
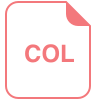
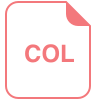
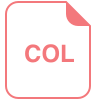
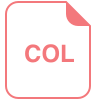
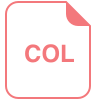
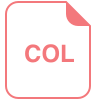





