人机操控平台:启动程序后,系统提供给用户一个操作界面,以便用户有效地操作游戏。 选择游戏难度:贪吃蛇游戏需要用户选择不同的难度,难度主要体现在蛇的移动速度上。 游戏操作和得分:使用上下左右键控制蛇的移动,寻找食物,食物分为普通食物和奖励性食物 吃普通食物蛇身会变长,分数增加,吃到奖励性食物,蛇身不变长,分数增加,但是奖励性食物 有时间限制。 游戏操作说明:系统给用户提供地图元素组成、操作规则等信息。C语言代码
时间: 2024-03-05 18:48:01 浏览: 8
以下是一个简单的C语言实现贪吃蛇游戏的代码,包括操作界面的绘制、游戏难度选择、游戏操作和得分,以及游戏操作说明的展示。
```
#include <stdio.h>
#include <conio.h>
#include <graphics.h>
#include <time.h>
#define SIZE 20 // 蛇身大小
#define WIDTH 640 // 界面宽度
#define HEIGHT 480 // 界面高度
// 蛇身结构体
struct snake {
int x;
int y;
struct snake *next;
};
// 食物结构体
struct food {
int x;
int y;
int type; // 0为普通食物,1为奖励性食物
time_t start_time; // 奖励性食物开始时间
};
// 绘制蛇身
void draw_snake(struct snake *head) {
struct snake *p = head;
while (p != NULL) {
rectangle(p->x, p->y, p->x + SIZE, p->y + SIZE);
p = p->next;
}
floodfill(head->x + SIZE / 2, head->y + SIZE / 2, WHITE);
}
// 绘制食物
void draw_food(struct food f) {
setfillstyle(SOLID_FILL, f.type == 0 ? GREEN : RED);
pieslice(f.x + SIZE / 2, f.y + SIZE / 2, 0, 360, SIZE / 2);
}
// 判断蛇是否吃到食物
int is_eat(struct snake *head, struct food f) {
return head->x == f.x && head->y == f.y;
}
// 生成食物
struct food generate_food() {
struct food f;
f.x = rand() % (WIDTH / SIZE) * SIZE;
f.y = rand() % (HEIGHT / SIZE) * SIZE;
f.type = rand() % 2;
f.start_time = time(NULL);
return f;
}
// 蛇移动
void move_snake(struct snake *head, int direction) {
struct snake *p = head;
int x = p->x, y = p->y;
while (p != NULL) {
if (p == head) {
switch (direction) {
case 0: // 上
p->y -= SIZE;
break;
case 1: // 下
p->y += SIZE;
break;
case 2: // 左
p->x -= SIZE;
break;
case 3: // 右
p->x += SIZE;
break;
}
} else {
int tmp_x = p->x, tmp_y = p->y;
p->x = x;
p->y = y;
x = tmp_x;
y = tmp_y;
}
p = p->next;
}
}
// 判断蛇是否撞墙或自身
int is_dead(struct snake *head) {
int x = head->x, y = head->y;
if (x < 0 || x >= WIDTH || y < 0 || y >= HEIGHT) {
return 1;
}
struct snake *p = head->next;
while (p != NULL) {
if (p->x == x && p->y == y) {
return 1;
}
p = p->next;
}
return 0;
}
// 游戏结束
void game_over(int score) {
cleardevice();
settextstyle(4, 0, 6);
setcolor(YELLOW);
outtextxy(WIDTH / 2 - 120, HEIGHT / 2 - 50, "GAME OVER");
char str[20];
sprintf(str, "SCORE: %d", score);
outtextxy(WIDTH / 2 - 80, HEIGHT / 2 + 50, str);
getch();
closegraph();
}
int main() {
initwindow(WIDTH, HEIGHT);
setbkcolor(BLACK);
cleardevice();
srand(time(NULL));
// 绘制操作说明
settextstyle(4, 0, 2);
setcolor(WHITE);
outtextxy(10, 10, "Press up/down/left/right to move.");
outtextxy(10, 30, "Eat green food to grow longer.");
outtextxy(10, 50, "Eat red food to get more score.");
outtextxy(10, 70, "Red food will disappear after 5 seconds.");
// 选择游戏难度
int speed;
printf("Please select the difficulty of the game (1-5): ");
scanf("%d", &speed);
speed = 6 - speed;
// 初始化蛇
struct snake *head = (struct snake *) malloc(sizeof(struct snake));
head->x = WIDTH / 2;
head->y = HEIGHT / 2;
head->next = NULL;
draw_snake(head);
// 初始化食物
struct food f = generate_food();
draw_food(f);
// 游戏开始
int direction = 3; // 初始方向为右
int score = 0;
while (1) {
// 移动蛇
move_snake(head, direction);
if (is_dead(head)) {
game_over(score);
return 0;
}
cleardevice();
draw_snake(head);
// 判断是否吃到食物
if (is_eat(head, f)) {
if (f.type == 0) { // 普通食物
struct snake *p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = (struct snake *) malloc(sizeof(struct snake));
p->next->x = p->x;
p->next->y = p->y;
p->next->next = NULL;
} else { // 奖励性食物
score += 10;
}
f = generate_food();
draw_food(f);
score += 5;
}
// 判断奖励性食物是否超时
if (f.type == 1 && time(NULL) - f.start_time >= 5) {
f = generate_food();
draw_food(f);
}
// 显示得分
char str[20];
sprintf(str, "SCORE: %d", score);
settextstyle(4, 0, 2);
setcolor(WHITE);
outtextxy(10, HEIGHT - 30, str);
// 控制蛇的移动速度
delay(speed);
// 监听键盘事件
if (kbhit()) {
char c = getch();
switch (c) {
case 'w':
case 'W':
case 72: // 上
if (direction != 1) {
direction = 0;
}
break;
case 's':
case 'S':
case 80: // 下
if (direction != 0) {
direction = 1;
}
break;
case 'a':
case 'A':
case 75: // 左
if (direction != 3) {
direction = 2;
}
break;
case 'd':
case 'D':
case 77: // 右
if (direction != 2) {
direction = 3;
}
break;
}
}
}
return 0;
}
```
相关推荐
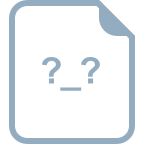
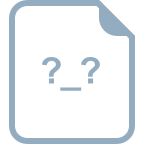
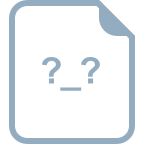














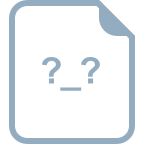