用python开发一个点菜菜单
时间: 2024-09-13 10:02:21 浏览: 36
使用Python开发一个简单的点菜菜单系统,你可以采用GUI库如Tkinter或wxPython来设计用户界面,同时结合字典或者列表数据结构来存储菜品信息。下面是一个基本的示例:
```python
import tkinter as tk
class MenuApp(tk.Tk):
def __init__(self):
super().__init__()
# 创建菜品字典
self.menu_items = {
"红烧肉": {"price": 30, "description": "经典的家常菜"},
"鱼香茄子": {"price": 25, "description": "酸甜可口"},
"宫保鸡丁": {"price": 28, "description": "辣味十足"}
}
# 初始化界面
self.init_ui()
def init_ui(self):
self.title("餐厅点菜系统")
# 菜品列表框
self.order_listbox = tk.Listbox(self)
for item in self.menu_items.keys():
self.order_listbox.insert(tk.END, item)
# 价格显示
self.price_label = tk.Label(self, text="总价: $0")
self.price_label.pack()
# 下单按钮
self.submit_button = tk.Button(self, text="下单", command=self.submit_order)
self.submit_button.pack()
def submit_order(self):
selected_item = self.order_listbox.get(tk.ACTIVE) # 获取选中的菜品
price = self.menu_items[selected_item]["price"] # 获取价格
total_price = int(self.price_label.cget("text").split("$")[1]) + price # 计算总价
self.price_label.config(text=f"总价: ${total_price}")
# 主程序
if __name__ == "__main__":
app = MenuApp()
app.mainloop()
```
在这个例子中,用户可以从列表框选择菜品,点击下单按钮会更新总价标签。这只是一个基础版本,实际应用可能需要添加搜索、删除、修改菜品功能,以及数据库持久化等。
**相关问题--:**
1. 如何增加用户输入价格的功能?
2. 如何实现菜品图片的展示?
3. 怎样将此点菜系统连接到后台数据库进行管理?
阅读全文
相关推荐
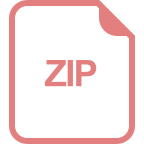
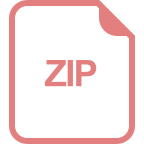
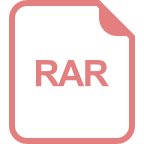
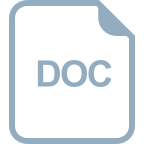

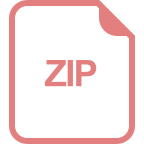
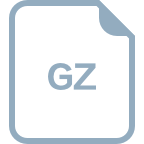
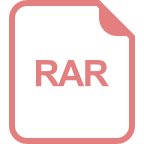
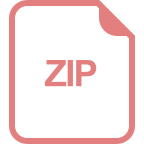
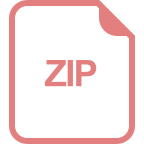
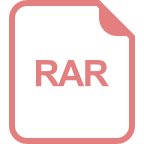
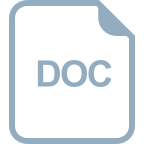
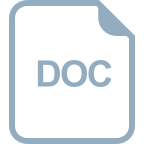
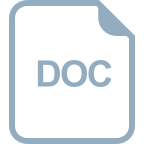
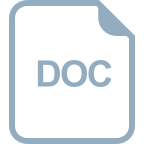
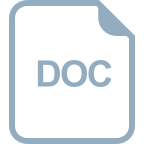



