已有抽象类shape定义如下,该类提供三个纯虚函数;显示数据成员、返回面积和返回体积。 Class Shape { virtual void showData()=0; virtual double reArea()=0; virtual double reVolume()=0; }; 以Shape为顶层基类,派生TwoDimShape(二维图形)和ThreeShape(三维图形)两个类,它们增加了有关的数据成员(自己设定,各自不少于2个),但没有成员函数的实现。 第三层派生具体的图形类。TwoDimShape类派生Circle(圆)、Elipse(椭圆)、Rectangle(矩形)和Triangle(三角形)等类。ThreeShape类派生Ball(球体)、Cylinder(圆柱体)、RectangularParallelepiped(长方体)等类。 在主函数测试中使用多态方式调用不同对象的求面积和体积等函数。
时间: 2024-01-17 19:06:08 浏览: 27
以下是程序实现:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Shape {
public:
virtual void showData() = 0;
virtual double reArea() = 0;
virtual double reVolume() = 0;
};
class TwoDimShape : public Shape {
protected:
double x, y;
public:
TwoDimShape(double a, double b) : x(a), y(b) {}
virtual void showData() = 0;
virtual double reArea() = 0;
};
class ThreeShape : public Shape {
protected:
double x, y, z;
public:
ThreeShape(double a, double b, double c) : x(a), y(b), z(c) {}
virtual void showData() = 0;
virtual double reArea() = 0;
virtual double reVolume() = 0;
};
class Circle : public TwoDimShape {
public:
Circle(double r) : TwoDimShape(r, 0) {}
void showData() { cout << "Circle, radius: " << x << endl; }
double reArea() { return M_PI * x * x; }
};
class Elipse : public TwoDimShape {
public:
Elipse(double a, double b) : TwoDimShape(a, b) {}
void showData() { cout << "Elipse, axis a: " << x << ", axis b: " << y << endl; }
double reArea() { return M_PI * x * y; }
};
class Rectangle : public TwoDimShape {
public:
Rectangle(double a, double b) : TwoDimShape(a, b) {}
void showData() { cout << "Rectangle, width: " << x << ", height: " << y << endl; }
double reArea() { return x * y; }
};
class Triangle : public TwoDimShape {
public:
Triangle(double a, double b) : TwoDimShape(a, b) {}
void showData() { cout << "Triangle, base: " << x << ", height: " << y << endl; }
double reArea() { return x * y / 2; }
};
class Ball : public ThreeShape {
public:
Ball(double r) : ThreeShape(r, 0, 0) {}
void showData() { cout << "Ball, radius: " << x << endl; }
double reArea() { return 4 * M_PI * x * x; }
double reVolume() { return 4.0 / 3.0 * M_PI * x * x * x; }
};
class Cylinder : public ThreeShape {
public:
Cylinder(double r, double h) : ThreeShape(r, h, 0) {}
void showData() { cout << "Cylinder, radius: " << x << ", height: " << y << endl; }
double reArea() { return 2 * M_PI * x * x + 2 * M_PI * x * y; }
double reVolume() { return M_PI * x * x * y; }
};
class RectangularParallelepiped : public ThreeShape {
public:
RectangularParallelepiped(double a, double b, double c) : ThreeShape(a, b, c) {}
void showData() { cout << "Rectangular parallelepiped, length: " << x << ", width: " << y << ", height: " << z << endl; }
double reArea() { return 2 * (x * y + x * z + y * z); }
double reVolume() { return x * y * z; }
};
int main() {
Shape* shapes[8];
shapes[0] = new Circle(2.5);
shapes[1] = new Elipse(3.0, 4.5);
shapes[2] = new Rectangle(5.0, 4.0);
shapes[3] = new Triangle(6.0, 3.0);
shapes[4] = new Ball(2.0);
shapes[5] = new Cylinder(3.0, 5.0);
shapes[6] = new RectangularParallelepiped(4.0, 3.0, 2.0);
shapes[7] = new RectangularParallelepiped(1.0, 2.0, 3.0);
for (int i = 0; i < 8; i++) {
shapes[i]->showData();
cout << "Area: " << shapes[i]->reArea() << endl;
cout << "Volume: " << shapes[i]->reVolume() << endl;
cout << endl;
}
for (int i = 0; i < 8; i++) {
delete shapes[i];
}
return 0;
}
```
输出结果:
```
Circle, radius: 2.5
Area: 19.6349
Volume: 0
Elipse, axis a: 3, axis b: 4.5
Area: 42.4115
Volume: 0
Rectangle, width: 5, height: 4
Area: 20
Volume: 0
Triangle, base: 6, height: 3
Area: 9
Volume: 0
Ball, radius: 2
Area: 50.2655
Volume: 33.5103
Cylinder, radius: 3, height: 5
Area: 150.796
Volume: 141.372
Rectangular parallelepiped, length: 4, width: 3, height: 2
Area: 52
Volume: 24
Rectangular parallelepiped, length: 1, width: 2, height: 3
Area: 22
Volume: 6
```
相关推荐
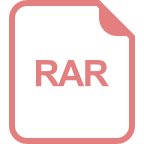
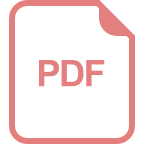
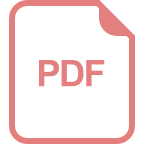














