使用python 将我输入的骨架图片里的骨架进行曲线拟合 批量图片输入和批量图片输出
时间: 2024-05-11 14:14:06 浏览: 42
这个任务可以使用OpenCV和Numpy库来完成。下面是一个基本的代码示例:
```python
import cv2
import numpy as np
import os
def curve_fitting(img):
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY_INV)
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_NONE)
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
roi = img[y:y + h, x:x + w]
curve = cv2.Canny(roi, 50, 150)
curve = cv2.cvtColor(curve, cv2.COLOR_GRAY2BGR)
curve = cv2.GaussianBlur(curve, (3, 3), 0)
curve = cv2.cvtColor(curve, cv2.COLOR_BGR2GRAY)
curve = cv2.threshold(curve, 0, 255, cv2.THRESH_BINARY)[1]
rows, cols = curve.shape[:2]
if rows > 0 and cols > 0:
pts = np.array(np.column_stack(np.where(curve > 0)), dtype=np.float32)
if len(pts) > 0:
pts[:, 0] = pts[:, 0] + x
pts[:, 1] = pts[:, 1] + y
curve = cv2.cvtColor(curve, cv2.COLOR_GRAY2BGR)
curve = cv2.polylines(curve, [np.int32(pts)], False, (0, 0, 255), 2)
img[y:y + h, x:x + w] = curve
return img
# 批量处理图片
input_dir = 'input_dir'
output_dir = 'output_dir'
if not os.path.exists(output_dir):
os.makedirs(output_dir)
for file in os.listdir(input_dir):
if file.endswith('.jpg'):
img = cv2.imread(os.path.join(input_dir, file))
img = curve_fitting(img)
cv2.imwrite(os.path.join(output_dir, file), img)
```
这个代码将会对指定目录下的所有.jpg文件进行骨架曲线拟合,并将结果保存到指定的输出目录。你可以根据需要调整代码中的参数、阈值等来获取更好的结果。
阅读全文
相关推荐



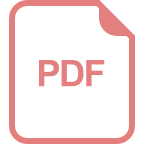
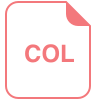
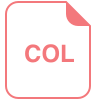
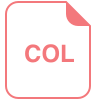
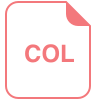


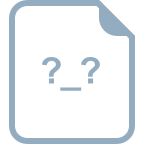
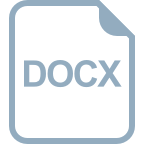