在WPF设计中有一个DataGrid表格,可以修改,共4行3列,其列的格式都为DataGridTextColumn,现在双击修改表中某个单元格数据之后,DataGrid表格数据自动刷新显示最新的修改后的数据,请给出完整的实例和代码
时间: 2023-11-22 17:55:49 浏览: 108
这里是一个简单的实例和代码,可以实现你所需的功能:
XAML代码:
```xml
<Window x:Class="DataGridUpdateOnDoubleClick.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="MainWindow" Height="350" Width="525">
<Grid>
<DataGrid x:Name="MyDataGrid" AutoGenerateColumns="False" IsReadOnly="False"
CellEditEnding="MyDataGrid_CellEditEnding">
<DataGrid.Columns>
<DataGridTextColumn Header="ID" Binding="{Binding ID}" />
<DataGridTextColumn Header="Name" Binding="{Binding Name}" />
<DataGridTextColumn Header="Age" Binding="{Binding Age}" />
</DataGrid.Columns>
</DataGrid>
</Grid>
</Window>
```
代码中绑定了一个名为`MyDataGrid`的DataGrid控件,包含3个列,分别是`ID`、`Name`和`Age`。同时设置了`AutoGenerateColumns`为`False`,这意味着我们必须手动添加列。`IsReadOnly`属性设置为`False`,允许我们在表格中编辑数据。`CellEditEnding`事件将在编辑单元格完成后触发。
C#代码:
```csharp
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Windows;
namespace DataGridUpdateOnDoubleClick
{
public partial class MainWindow : Window
{
private ObservableCollection<Person> persons = new ObservableCollection<Person>();
public MainWindow()
{
InitializeComponent();
// 添加一些测试数据
persons.Add(new Person { ID = 1, Name = "张三", Age = 20 });
persons.Add(new Person { ID = 2, Name = "李四", Age = 25 });
persons.Add(new Person { ID = 3, Name = "王五", Age = 30 });
// 将数据绑定到 DataGrid 控件上
MyDataGrid.ItemsSource = persons;
}
private void MyDataGrid_CellEditEnding(object sender, DataGridCellEditEndingEventArgs e)
{
// 当单元格编辑完成后,更新数据
if (e.EditAction == DataGridEditAction.Commit)
{
var person = e.Row.DataContext as Person;
if (person != null)
{
var column = e.Column as DataGridTextColumn;
if (column != null)
{
var binding = column.Binding as System.Windows.Data.Binding;
if (binding != null)
{
var property = binding.Path.Path;
if (property == "ID")
{
int id;
if (int.TryParse(((TextBox)e.EditingElement).Text, out id))
{
person.ID = id;
}
}
else if (property == "Name")
{
person.Name = ((TextBox)e.EditingElement).Text;
}
else if (property == "Age")
{
int age;
if (int.TryParse(((TextBox)e.EditingElement).Text, out age))
{
person.Age = age;
}
}
else
{
// 其他列不做处理
}
}
}
}
}
}
}
public class Person : INotifyPropertyChanged
{
private int id;
public int ID
{
get { return id; }
set
{
if (id != value)
{
id = value;
OnPropertyChanged("ID");
}
}
}
private string name;
public string Name
{
get { return name; }
set
{
if (name != value)
{
name = value;
OnPropertyChanged("Name");
}
}
}
private int age;
public int Age
{
get { return age; }
set
{
if (age != value)
{
age = value;
OnPropertyChanged("Age");
}
}
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string propertyName)
{
var handler = PropertyChanged;
if (handler != null)
{
handler(this, new PropertyChangedEventArgs(propertyName));
}
}
}
}
```
`Person`类实现`INotifyPropertyChanged`接口,用于在属性值变化时通知界面更新数据。`MainWindow`类中的构造函数初始化了表格数据和绑定数据。`MyDataGrid_CellEditEnding`事件处理函数在单元格编辑完成后更新数据。由于每个单元格都有自己的绑定,因此需要根据绑定的属性名称来更新对应的数据。
在这个示例中,我们只处理了`ID`、`Name`和`Age`三列,其他列可以按照类似的方式进行处理。
阅读全文
相关推荐
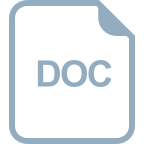
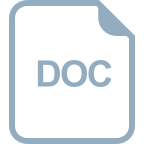
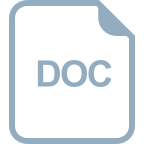


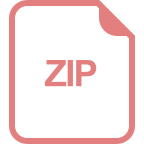












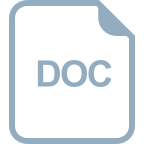