用 VHDL 编写一个 8路彩灯控制程序,要求彩灯有以下3种演示花型。 (1)8路彩灯同时亮灭; (2)从左至右逐个亮(每次只有1路亮); (3)从两边往中间逐个亮:全灭:从中间往两头逐个亮:全灭;
时间: 2024-11-03 10:19:13 浏览: 75
在VHDL中,我们可以使用组合逻辑和计数器来实现这个8路彩灯控制程序。这里是一个基本的设计思路:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity LEDPatternController is
Port (
Enable : in STD_LOGIC; -- 全局控制信号
PatternSelect : in STD_LOGIC_VECTOR(2 downto 0); -- 选择模式
RGB : in STD_LOGIC_VECTOR(7 downto 0); -- 彩灯RGB值
LEDs : out STD_LOGIC_VECTOR(7 downto 0) -- 8路LED输出
);
end LEDPatternController;
architecture Behavioral of LEDPatternController is
constant NUM_LEDS : integer := 8;
type LEDPattern is (AllOn, Sequential, Bounce);
-- 表示当前操作的局部信号
signal CurrentPattern : LEDPattern := AllOn;
signal LEDIndex : integer range 0 to NUM_LEDS - 1 := 0;
begin
process(Enable, PatternSelect)
begin
case PatternSelect is
when "000" => -- 全部同时亮灭
if Enable = '1' then
LEDs <= RGB;
else
LEDs <= 'Z'; -- 黑色,关闭所有LEDs
end if;
when "001" => -- 逐个从左至右亮
if Enable = '1' then
LEDs <= RGB(LEDIx) & 'Z' & 'Z'(others => 'Z');
LEDIndex <= LEDIndex + 1;
end if;
when "010" | "011" => -- 从两边往中间逐亮和逐暗
-- ...此处详细写出这部分逻辑...
end case;
-- 当LEDIndex超过范围或需要切换模式时,更新CurrentPattern和LEDIndex
if CurrentPattern = Bounce and LEDIndex = NUM_LEDS / 2 or
LEDIndex >= NUM_LEDS then
CurrentPattern := AllOn;
LEDIndex := 0;
end if;
end process;
-- 当需要改变模式时,更新PatternSelect
process(PatternSelect)
begin
if rising_edge(PatternSelect) then
if PatternSelect = "010" then
CurrentPattern := Sequential;
elsif PatternSelect = "011" then
CurrentPattern := Bounce;
end if;
end if;
end process;
end Behavioral;
-- 相关问题--
-- 1. 这个设计如何处理模式之间的转换?
-- 2. 如何实现Bounce模式(闪烁效果)?
-- 3. 能否添加一个延时机制来增加闪烁效果?
```
上述代码给出了一个基础框架,实际的Bounce模式(闪烁效果)可能需要使用定时器或者异步触发器来控制LED的开关,以及适当的延时。请注意,完整的Bounce模式实现将涉及到复杂的时序逻辑和可能的计数器结构。
阅读全文
相关推荐
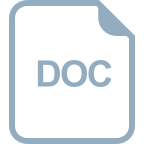
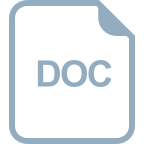
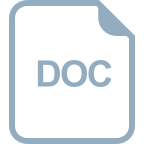

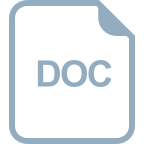
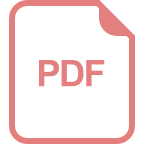
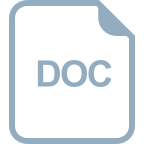
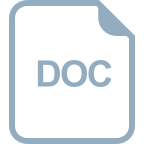
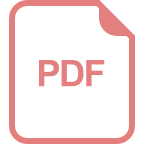
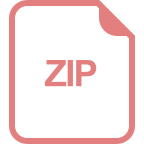
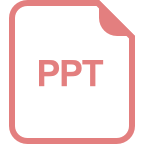
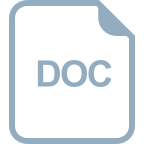
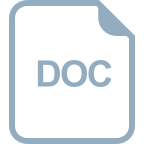
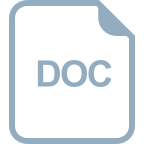
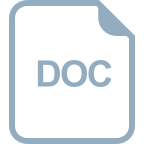
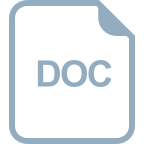


