c++通讯录程序设计
时间: 2024-04-27 21:15:58 浏览: 102
C++通讯录程序设计是一个常见的编程练习项目,它可以帮助你学习和实践C++的基本概念和技巧。下面是一个简单的C++通讯录程序设计的示例:
1. 定义联系人类(Contact):联系人类可以包含姓名、电话号码等信息,并提供相应的成员函数来设置和获取这些信息。
2. 定义通讯录类(AddressBook):通讯录类可以包含多个联系人对象,并提供相应的成员函数来添加、删除、查找和显示联系人信息。
3. 实现通讯录功能:在主函数中,创建一个通讯录对象,并通过菜单选项来执行不同的操作,如添加联系人、删除联系人、查找联系人、显示所有联系人等。
下面是一个简单的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Contact {
private:
string name;
string phoneNumber;
public:
Contact(string name, string phoneNumber) {
this->name = name;
this->phoneNumber = phoneNumber;
}
string getName() {
return name;
}
string getPhoneNumber() {
return phoneNumber;
}
};
class AddressBook {
private:
vector<Contact> contacts;
public:
void addContact(Contact contact) {
contacts.push_back(contact);
}
void removeContact(string name) {
for (int i = 0; i < contacts.size(); i++) {
if (contacts[i].getName() == name) {
contacts.erase(contacts.begin() + i);
break;
}
}
}
Contact findContact(string name) {
for (int i = 0; i < contacts.size(); i++) {
if (contacts[i].getName() == name) {
return contacts[i];
}
}
return Contact("", "");
}
void displayContacts() {
for (int i = 0; i < contacts.size(); i++) {
cout << "Name: " << contacts[i].getName() << ", Phone Number: " << contacts[i].getPhoneNumber() << endl;
}
}
};
int main() {
AddressBook addressBook;
while (true) {
cout << "Menu:" << endl;
cout << "1. Add Contact" << endl;
cout << "2. Remove Contact" << endl;
cout << "3. Find Contact" << endl;
cout << "4. Display Contacts" << endl;
cout << "5. Exit" << endl;
int choice;
cin >> choice;
if (choice == 1) {
string name, phoneNumber;
cout << "Enter name: ";
cin >> name;
cout << "Enter phone number: ";
cin >> phoneNumber;
Contact contact(name, phoneNumber);
addressBook.addContact(contact);
} else if (choice == 2) {
string name;
cout << "Enter name: ";
cin >> name;
addressBook.removeContact(name);
} else if (choice == 3) {
string name;
cout << "Enter name: ";
cin >> name;
Contact contact = addressBook.findContact(name);
if (contact.getName() != "") {
cout << "Name: " << contact.getName() << ", Phone Number: " << contact.getPhoneNumber() << endl;
} else {
cout << "Contact not found." << endl;
}
} else if (choice == 4) {
addressBook.displayContacts();
} else if (choice == 5) {
break;
} else {
cout << "Invalid choice. Please try again." << endl;
}
}
return 0;
}
```
这个示例程序实现了一个简单的通讯录功能,可以通过菜单选项来添加、删除、查找和显示联系人信息。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
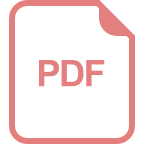
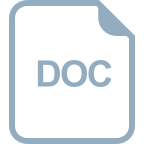
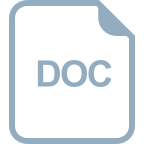
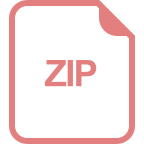
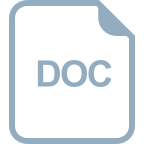
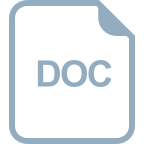
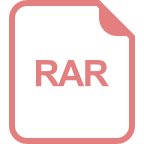
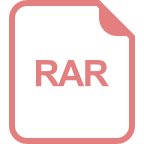
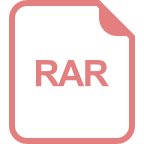
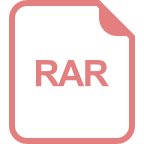
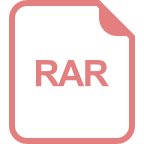
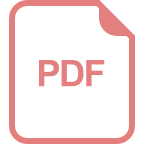
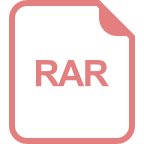
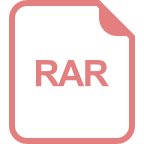
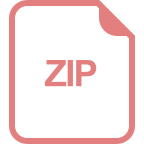
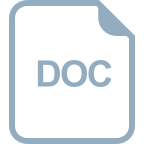