通过遗传算法求函数F(x)=xsin(10π*x)+2.0 x∈[-1,2]的最大值。
时间: 2023-10-07 16:12:18 浏览: 119
好的,这是一道优化问题,可以使用遗传算法进行求解。下面是具体的步骤:
1. 初始化种群:随机生成一定数量的个体,每个个体都是一个[-1,2]范围内的实数。
2. 评估个体适应度:将每个个体带入函数F(x)中计算适应度值。
3. 选择:使用轮盘赌选择法从种群中选择一定数量的优秀个体,将其作为下一代种群的父代。
4. 交叉:使用单点交叉或多点交叉对父代进行交叉操作,生成子代。
5. 变异:对子代进行变异操作,随机改变其中的某些基因值。
6. 更新种群:将子代和父代合并,得到下一代种群。
7. 判断停止条件:如果达到了指定的停止条件(如达到最大迭代次数或适应度值已经趋于稳定),则停止迭代,输出最优解。
8. 回到第2步,继续迭代,直到满足停止条件为止。
通过遗传算法的迭代,可以逐步逼近函数的最大值。注意,遗传算法的求解结果不一定是全局最优解,但可以得到较为优秀的近似解。
相关问题
用Python通过遗传算法求函数F(x)=xsin(10π*x)+2.0 x∈[-1,2]的最大值。
好的,下面是一个简单的Python代码实现,使用遗传算法求解函数F(x)的最大值:
```python
import random
import math
# 定义函数F(x)
def F(x):
return x * math.sin(10 * math.pi * x) + 2.0
# 定义遗传算法参数
POP_SIZE = 50 # 种群大小
GENE_LENGTH = 20 # 基因长度
CROSS_RATE = 0.8 # 交叉概率
MUTATE_RATE = 0.01 # 变异概率
N_GENERATIONS = 200 # 迭代次数
# 初始化种群
pop = [[random.uniform(-1, 2) for _ in range(GENE_LENGTH)] for _ in range(POP_SIZE)]
# 遗传算法迭代
for generation in range(N_GENERATIONS):
# 计算每个个体的适应度
fitness = [F(ind) for ind in pop]
# 选择优秀个体作为父代
parents = []
for _ in range(POP_SIZE):
idx1, idx2 = random.randint(0, POP_SIZE-1), random.randint(0, POP_SIZE-1)
if fitness[idx1] > fitness[idx2]:
parents.append(pop[idx1])
else:
parents.append(pop[idx2])
# 交叉操作
for child in parents:
if random.random() < CROSS_RATE:
idx = random.randint(0, POP_SIZE-1)
cross_points = random.randint(0, GENE_LENGTH)
child[cross_points:] = pop[idx][cross_points:]
# 变异操作
for child in parents:
if random.random() < MUTATE_RATE:
mutate_point = random.randint(0, GENE_LENGTH-1)
child[mutate_point] += random.uniform(-0.1, 0.1)
# 更新种群
pop = parents
# 输出最优解
best_ind = pop[0]
best_fitness = F(best_ind)
for ind in pop:
if F(ind) > best_fitness:
best_ind = ind
best_fitness = F(ind)
print("最优解:", best_ind)
print("最大值:", best_fitness)
```
这段代码使用了Python的随机数生成函数,以及math库中的数学函数。遗传算法部分的实现也比较简单,主要包括种群初始化、适应度计算、选择、交叉、变异和种群更新等步骤。通过迭代,可以逐步逼近函数F(x)的最大值。
用Python通过遗传算法求函数F(x)=xsin(10π*x)+2.0 x∈[-1,2]的最大值,并包含图像。
好的,我可以帮你解决这个问题。遗传算法是一种优化算法,可以用来求解复杂的函数问题。我们可以使用Python的遗传算法库DEAP来实现这个问题。
首先,需要安装DEAP库。可以使用以下命令进行安装:
```
pip install deap
```
接下来,我们需要定义问题的适应度函数。对于本问题,适应度函数为F(x)=xsin(10πx)+2.0,我们需要求的是这个函数的最大值。因此,适应度函数应该返回函数值的相反数(因为DEAP库的优化算法是寻找最小值)。
```python
import math
from deap import base, creator, tools
# 定义适应度函数
def evaluate(individual):
x = individual[0]
return -1 * (x * math.sin(10 * math.pi * x) + 2.0),
# 创建适应度函数适应度类
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
# 创建个体类
creator.create("Individual", list, fitness=creator.FitnessMax)
```
接下来,我们需要定义遗传算法的参数。这些参数包括种群大小、交叉率、变异率等。
```python
# 定义遗传算法参数
POPULATION_SIZE = 50
P_CROSSOVER = 0.9
P_MUTATION = 0.1
MAX_GENERATIONS = 50
HALL_OF_FAME_SIZE = 1
toolbox = base.Toolbox()
# 定义变量范围
toolbox.register("attr_float", random.uniform, -1, 2)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_float, n=1)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# 定义交叉函数
toolbox.register("mate", tools.cxBlend, alpha=0.2)
# 定义变异函数
toolbox.register("mutate", tools.mutGaussian, mu=0, sigma=0.1, indpb=0.1)
toolbox.register("select", tools.selTournament, tournsize=3)
toolbox.register("evaluate", evaluate)
```
现在我们可以开始遗传算法的主循环。在每一代中,我们使用选择、交叉和变异操作来生成新的种群,并使用适应度函数来评估每个个体的适应度。在每一代中,我们都会选择适应度最好的个体,并将其添加到名人堂中。
```python
import random
random.seed(42)
population = toolbox.population(n=POPULATION_SIZE)
hall_of_fame = tools.HallOfFame(HALL_OF_FAME_SIZE)
stats = tools.Statistics(lambda ind: ind.fitness.values)
stats.register("avg", tools.mean)
stats.register("min", tools.min)
logbook = tools.Logbook()
logbook.header = ["generation", "evaluations"] + stats.fields
for generation in range(MAX_GENERATIONS):
offspring = algorithms.varOr(population, toolbox, lambda_=POPULATION_SIZE, cxpb=P_CROSSOVER, mutpb=P_MUTATION)
fits = toolbox.map(toolbox.evaluate, offspring)
for fit, ind in zip(fits, offspring):
ind.fitness.values = fit
population = toolbox.select(offspring, k=len(population))
hall_of_fame.update(population)
record = stats.compile(population)
logbook.record(evaluations=len(population), generation=generation, **record)
print(logbook.stream)
best = hall_of_fame.items[0]
print("Best individual is", best, "with fitness", best.fitness.values[0])
```
最后,我们可以使用Matplotlib库绘制函数的图像和最优解的位置。
```python
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-1, 2, 200)
y = x * np.sin(10 * np.pi * x) + 2.0
plt.plot(x, y)
plt.plot(best, best.fitness.values[0], 'go')
plt.show()
```
完整代码如下:
```python
import math
import random
import numpy as np
import matplotlib.pyplot as plt
from deap import base, creator, tools, algorithms
# 定义适应度函数
def evaluate(individual):
x = individual[0]
return -1 * (x * math.sin(10 * math.pi * x) + 2.0),
# 创建适应度函数适应度类
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
# 创建个体类
creator.create("Individual", list, fitness=creator.FitnessMax)
# 定义遗传算法参数
POPULATION_SIZE = 50
P_CROSSOVER = 0.9
P_MUTATION = 0.1
MAX_GENERATIONS = 50
HALL_OF_FAME_SIZE = 1
toolbox = base.Toolbox()
# 定义变量范围
toolbox.register("attr_float", random.uniform, -1, 2)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_float, n=1)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# 定义交叉函数
toolbox.register("mate", tools.cxBlend, alpha=0.2)
# 定义变异函数
toolbox.register("mutate", tools.mutGaussian, mu=0, sigma=0.1, indpb=0.1)
toolbox.register("select", tools.selTournament, tournsize=3)
toolbox.register("evaluate", evaluate)
random.seed(42)
population = toolbox.population(n=POPULATION_SIZE)
hall_of_fame = tools.HallOfFame(HALL_OF_FAME_SIZE)
stats = tools.Statistics(lambda ind: ind.fitness.values)
stats.register("avg", tools.mean)
stats.register("min", tools.min)
logbook = tools.Logbook()
logbook.header = ["generation", "evaluations"] + stats.fields
for generation in range(MAX_GENERATIONS):
offspring = algorithms.varOr(population, toolbox, lambda_=POPULATION_SIZE, cxpb=P_CROSSOVER, mutpb=P_MUTATION)
fits = toolbox.map(toolbox.evaluate, offspring)
for fit, ind in zip(fits, offspring):
ind.fitness.values = fit
population = toolbox.select(offspring, k=len(population))
hall_of_fame.update(population)
record = stats.compile(population)
logbook.record(evaluations=len(population), generation=generation, **record)
print(logbook.stream)
best = hall_of_fame.items[0]
print("Best individual is", best, "with fitness", best.fitness.values[0])
# 绘制函数图像和最优解的位置
x = np.linspace(-1, 2, 200)
y = x * np.sin(10 * np.pi * x) + 2.0
plt.plot(x, y)
plt.plot(best, best.fitness.values[0], 'go')
plt.show()
```
阅读全文
相关推荐






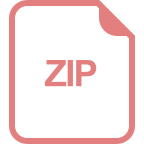





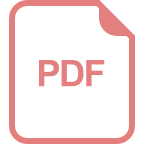