arr = np.expand_dims(arr, axis=-1)
时间: 2023-11-29 12:21:21 浏览: 149
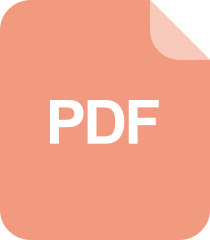
从numpy数组中取出满足条件的元素示例
这行代码使用了NumPy库的expand_dims函数,将数组arr在最后一个维度(axis=-1)上增加了一个维度。例如,如果arr原本的shape为(3, 4),那么执行这行代码后,arr的shape会变为(3, 4, 1)。这个操作通常用于将一维数组转换成二维数组,或者将二维数组转换成三维数组等等。这个操作在机器学习中也经常用到,例如在图像处理中,将二维图像转换成三维张量。
阅读全文
相关推荐
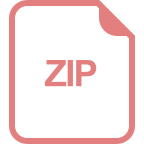
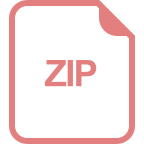














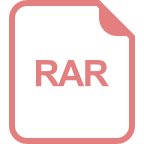
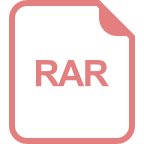