C++ 将成绩排序并将结果写入txt文件中
时间: 2023-09-20 09:02:59 浏览: 116
好的,您可以使用以下代码来实现:
```c++
#include <iostream>
#include <fstream>
#include <algorithm>
#include <vector>
using namespace std;
struct Student {
string name;
int score;
};
bool compare(Student a, Student b) {
return a.score > b.score;
}
int main() {
vector<Student> students;
ifstream infile("input.txt");
if (!infile) {
cout << "Error opening file!" << endl;
return 0;
}
string name;
int score;
while (infile >> name >> score) {
Student student = {name, score};
students.push_back(student);
}
infile.close();
sort(students.begin(), students.end(), compare);
ofstream outfile("output.txt");
if (!outfile) {
cout << "Error opening file!" << endl;
return 0;
}
for (int i = 0; i < students.size(); i++) {
outfile << students[i].name << " " << students[i].score << endl;
}
outfile.close();
return 0;
}
```
这个程序会从名为 `input.txt` 的文件中读取学生的姓名和成绩,将它们存储到 `vector` 中。然后使用 `sort` 函数将学生按成绩从高到低排序,最后将排好序的学生写入到名为 `output.txt` 的文件中。
注意,您需要在程序运行前创建 `input.txt` 文件,并在其中按照以下格式输入学生的姓名和成绩:
```
张三 90
李四 85
王五 92
```
输出的 `output.txt` 文件将按照以下格式进行输出:
```
王五 92
张三 90
李四 85
```
希望对您有所帮助!
阅读全文
相关推荐
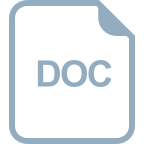
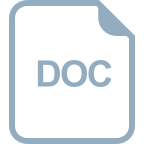
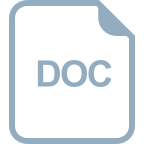

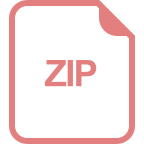

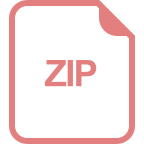
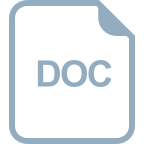
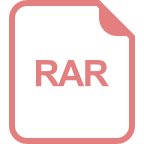









