用 python 画机器人
时间: 2023-07-04 13:28:20 浏览: 161
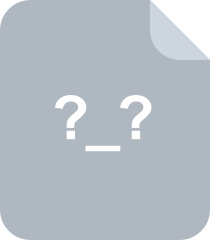
机器人设计(python)
下面是一个用 Python 和 Matplotlib 库绘制机器人的示例代码。
```
import matplotlib.pyplot as plt
# 定义机器人的关节角度
thetas = [0, 0.5, 1.0, 1.5, 2.0, 2.5]
# 定义机器人各个关节的长度
l1 = 1
l2 = 0.5
l3 = 1
l4 = 0.5
# 计算机器人末端的坐标
x = l1 * np.cos(thetas[0]) + l2 * np.cos(thetas[0] + thetas[1]) + l3 * np.cos(thetas[0] + thetas[1] + thetas[2]) + l4 * np.cos(thetas[0] + thetas[1] + thetas[2] + thetas[3])
y = l1 * np.sin(thetas[0]) + l2 * np.sin(thetas[0] + thetas[1]) + l3 * np.sin(thetas[0] + thetas[1] + thetas[2]) + l4 * np.sin(thetas[0] + thetas[1] + thetas[2] + thetas[3])
# 绘制机器人关节
fig, ax = plt.subplots()
ax.plot([0, l1 * np.cos(thetas[0])], [0, l1 * np.sin(thetas[0])], 'bo-', linewidth=2)
ax.plot([l1 * np.cos(thetas[0]), l1 * np.cos(thetas[0]) + l2 * np.cos(thetas[0] + thetas[1])], [l1 * np.sin(thetas[0]), l1 * np.sin(thetas[0]) + l2 * np.sin(thetas[0] + thetas[1])], 'ro-', linewidth=2)
ax.plot([l1 * np.cos(thetas[0]) + l2 * np.cos(thetas[0] + thetas[1]), l1 * np.cos(thetas[0]) + l2 * np.cos(thetas[0] + thetas[1]) + l3 * np.cos(thetas[0] + thetas[1] + thetas[2])], [l1 * np.sin(thetas[0]) + l2 * np.sin(thetas[0] + thetas[1]), l1 * np.sin(thetas[0]) + l2 * np.sin(thetas[0] + thetas[1]) + l3 * np.sin(thetas[0] + thetas[1] + thetas[2])], 'go-', linewidth=2)
ax.plot([l1 * np.cos(thetas[0]) + l2 * np.cos(thetas[0] + thetas[1]) + l3 * np.cos(thetas[0] + thetas[1] + thetas[2]), l1 * np.cos(thetas[0]) + l2 * np.cos(thetas[0] + thetas[1]) + l3 * np.cos(thetas[0] + thetas[1] + thetas[2]) + l4 * np.cos(thetas[0] + thetas[1] + thetas[2] + thetas[3])], [l1 * np.sin(thetas[0]) + l2 * np.sin(thetas[0] + thetas[1]) + l3 * np.sin(thetas[0] + thetas[1] + thetas[2]), l1 * np.sin(thetas[0]) + l2 * np.sin(thetas[0] + thetas[1]) + l3 * np.sin(thetas[0] + thetas[1] + thetas[2]) + l4 * np.sin(thetas[0] + thetas[1] + thetas[2] + thetas[3])], 'yo-', linewidth=2)
# 绘制机器人末端
ax.plot(x, y, 'ro', markersize=10)
# 设置坐标轴范围
ax.set_xlim([-3, 3])
ax.set_ylim([-3, 3])
# 显示图像
plt.show()
```
这个示例代码绘制了一个由四个关节组成的机器人,根据不同的关节角度计算机器人末端的坐标,并将机器人的关节和末端绘制出来。你可以根据自己的需求修改关节的数量、长度和角度,来绘制不同形状的机器人。
阅读全文
相关推荐
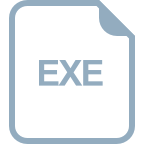


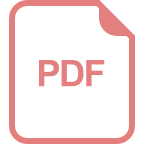

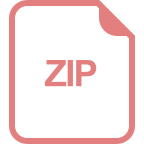
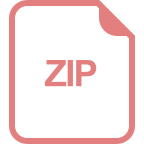
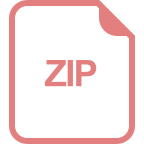
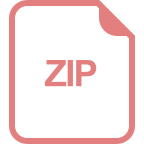
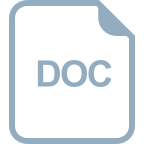
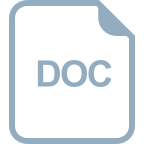
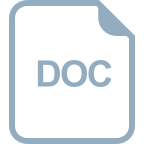


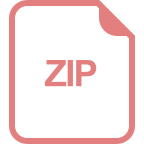