cnn与gnn相结合的图像分类
时间: 2023-08-22 09:10:11 浏览: 77
将CNN和GNN相结合的图像分类方法通常被称为图卷积神经网络(GCN)。以下是一种常见的CNN-GCN结合的图像分类方法:
1. 使用CNN提取图像特征。
2. 将CNN提取的特征矩阵作为图像的邻接矩阵,并使用GNN进行图卷积。
3. 在GNN中,每个节点表示CNN中提取的特征,每个边表示两个特征之间的关系。节点的标签是图像的类别标签。
4. 使用图分类算法(如图形卷积网络)对GNN中的节点进行分类,以确定图像的类别。
以下是一个简单的Python代码示例,演示如何使用CNN和GCN相结合进行图像分类:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
import torch.optim as optim
from torch_geometric.nn import GCNConv
from torch_geometric.data import Data
# CNN模型
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(3, 6, 5)
self.pool = nn.MaxPool2d(2, 2)
self.conv2 = nn.Conv2d(6, 16, 5)
self.fc1 = nn.Linear(16 * 5 * 5, 120)
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 10)
def forward(self, x):
x = self.pool(F.relu(self.conv1(x)))
x = self.pool(F.relu(self.conv2(x)))
x = x.view(-1, 16 * 5 * 5)
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = self.fc3(x)
return x
# GCN模型
class GCNNet(nn.Module):
def __init__(self):
super(GCNNet, self).__init__()
self.conv1 = GCNConv(16 * 5 * 5, 32)
self.conv2 = GCNConv(32, 64)
self.fc1 = nn.Linear(64, 10)
def forward(self, x, edge_index):
x = F.relu(self.conv1(x, edge_index))
x = F.relu(self.conv2(x, edge_index))
x = torch.mean(x, dim=0)
x = self.fc1(x)
return F.log_softmax(x, dim=1)
# 加载数据
train_dataset = torchvision.datasets.CIFAR10(root='./data', train=True, download=True, transform=transform)
test_dataset = torchvision.datasets.CIFAR10(root='./data', train=False, download=True, transform=transform)
train_loader = torch.utils.data.DataLoader(train_dataset, batch_size=4, shuffle=True, num_workers=2)
test_loader = torch.utils.data.DataLoader(test_dataset, batch_size=4, shuffle=False, num_workers=2)
# 训练CNN模型
cnn_net = Net()
optimizer = optim.SGD(cnn_net.parameters(), lr=0.001, momentum=0.9)
criterion = nn.CrossEntropyLoss()
for epoch in range(10):
running_loss = 0.0
for i, data in enumerate(train_loader, 0):
inputs, labels = data
optimizer.zero_grad()
outputs = cnn_net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
if i % 2000 == 1999:
print('[%d, %5d] loss: %.3f' % (epoch + 1, i + 1, running_loss / 2000))
running_loss = 0.0
# 获取CNN模型的特征矩阵
cnn_net.eval()
features = []
for data in train_loader:
inputs, labels = data
outputs = cnn_net(inputs)
features.append(outputs.detach().numpy())
features = np.vstack(features)
# 构建图并训练GCN模型
edge_index = torch.tensor([[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]] * 5000, dtype=torch.long)
edge_index = edge_index.transpose(0, 1).contiguous().view(-1, 2).t()
features = torch.tensor(features, dtype=torch.float)
labels = torch.tensor(train_dataset.targets, dtype=torch.long)
data = Data(x=features, edge_index=edge_index, y=labels)
gcn_net = GCNNet()
optimizer = optim.Adam(gcn_net.parameters(), lr=0.01, weight_decay=5e-4)
criterion = nn.CrossEntropyLoss()
for epoch in range(200):
gcn_net.train()
optimizer.zero_grad()
output = gcn_net(data.x, data.edge_index)
loss = criterion(output[data.train_mask], data.y[data.train_mask])
loss.backward()
optimizer.step()
print('Epoch %d | Loss: %.4f' % (epoch + 1, loss.item()))
# 测试模型
gcn_net.eval()
correct = 0
total = 0
with torch.no_grad():
for data in test_loader:
images, labels = data
outputs = cnn_net(images)
features = torch.tensor(outputs.detach().numpy(), dtype=torch.float)
data = Data(x=features, edge_index=edge_index)
output = gcn_net(data.x, data.edge_index)
_, predicted = torch.max(output.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print('Accuracy of the network on the 10000 test images: %d %%' % (100 * correct / total))
```
在这个示例中,我们使用PyTorch实现了一个简单的CNN模型和一个GCN模型,并将它们结合起来进行图像分类。首先,我们使用CNN提取图像特征。然后,我们将CNN提取的特征矩阵作为图像的邻接矩阵,并使用GNN进行图卷积。最后,我们使用图分类算法(在这种情况下是图形卷积网络)对GNN中的节点进行分类,以确定图像的类别。
相关推荐
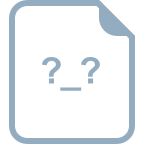
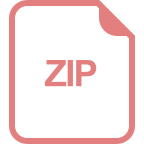














