unity 加锁单例基类
时间: 2023-09-20 11:13:32 浏览: 184
在 Unity 中,可以通过以下方式实现加锁单例基类:
```csharp
public class Singleton<T> where T : class
{
private static object _lock = new object();
private static T _instance;
public static T Instance
{
get
{
lock (_lock)
{
if (_instance == null)
{
_instance = Activator.CreateInstance(typeof(T), true) as T;
}
return _instance;
}
}
}
protected Singleton() { }
}
```
此基类使用泛型,可以方便地创建任何类型的单例。在 Instance 属性中,使用 lock 语句以确保线程安全。如果 _instance 为 null,则使用 Activator.CreateInstance 创建新对象。注意,创建对象的构造函数必须为受保护或私有,以确保只有单例基类可以创建新实例。
相关问题
unity 泛型单例模式
在Unity中,泛型单例模式是一种常用的设计模式,用于创建单例的管理类。通过继承一个泛型的基类Singleton<T>,我们可以省去重复编写单例模式的代码。[2]
在这个泛型基类中,我们定义了一个静态的Instance属性,用于获取单例的实例。在Awake方法中,我们判断如果已经存在一个实例,则销毁当前的游戏对象,否则将当前的实例赋值给instance。这样就保证了每个继承自Singleton<T>的类都只有一个实例。[2]
使用泛型单例模式时,只需要通过Singleton<T>.Instance来获取对应类的单例实例。比如,如果我们有一个名为PlayerCtrl的类,我们可以通过Singleton<PlayerCtrl>.Instance来获取PlayerCtrl类的单例。[3]
这种泛型单例模式的设计可以大大简化我们在Unity开发中创建管理类的过程,避免了重复编写单例模式的代码,提高了开发效率。
unity伪单例模式的写法
在 Unity 中实现伪单例模式,可以通过以下步骤进行:
1. 创建一个公共静态变量 instance,用于存储单例对象。
2. 在 Awake() 函数中判断 instance 是否为空,如果为空,则将当前对象赋值给 instance。
3. 在 Start() 函数中判断 instance 是否为空,如果为空,则销毁当前对象。
4. 在其他脚本中调用单例对象时,可以通过类名.instance的方式获取单例对象。
下面是示例代码:
```
public class Singleton : MonoBehaviour
{
public static Singleton instance;
private void Awake()
{
if (instance == null)
{
instance = this;
}
}
private void Start()
{
if (instance == null)
{
Destroy(gameObject);
}
}
}
```
在其他脚本中获取单例对象的方式如下:
```
Singleton singleton = Singleton.instance;
```
阅读全文
相关推荐
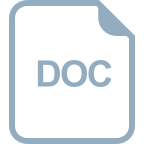
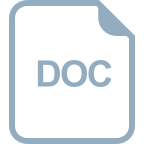
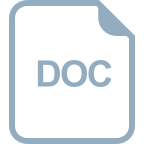
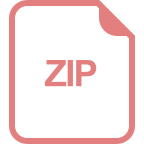
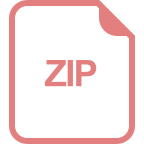
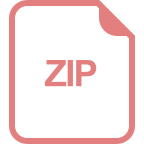




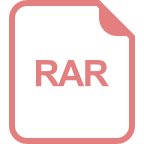
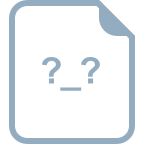
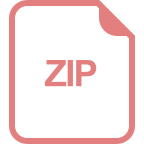
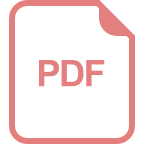

