Python对图像进行旋转,旋转后图像大小不变
时间: 2024-05-07 17:23:02 浏览: 143
要实现图像旋转后大小不变,可以使用OpenCV库中的函数cv2.warpAffine()。该函数可以对图像进行仿射变换,包括旋转、平移、缩放等操作。以下是一个示例代码:
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 获取图像中心点坐标
height, width = img.shape[:2]
center = (width/2, height/2)
# 设置旋转角度和缩放比例
angle = 45
scale = 1
# 计算变换矩阵
M = cv2.getRotationMatrix2D(center, angle, scale)
# 进行仿射变换
img_rotated = cv2.warpAffine(img, M, (width, height))
# 显示旋转后的图像
cv2.imshow('Rotated Image', img_rotated)
cv2.waitKey(0)
```
其中,cv2.getRotationMatrix2D()函数用于计算变换矩阵,参数包括旋转中心点、旋转角度和缩放比例;cv2.warpAffine()函数用于对图像进行仿射变换,参数包括变换矩阵和输出图像大小。
相关问题
python使用cv2库旋转图像,保持图像大小不变
可以使用cv2.warpAffine函数来实现图像旋转,同时保持图像大小不变。具体的实现步骤如下:
1. 计算图像中心点坐标
```python
(h, w) = image.shape[:2]
center = (w // 2, h // 2)
```
2. 构造旋转矩阵
```python
M = cv2.getRotationMatrix2D(center, angle, scale=1.0)
```
其中,angle是旋转角度,scale是缩放比例(默认为1.0)。
3. 对图像进行旋转
```python
rotated = cv2.warpAffine(image, M, (w, h))
```
这里的输出rotated即为旋转后的图像,它的大小与原图像相同。
完整代码如下:
```python
import cv2
# 加载图像
image = cv2.imread("image.jpg")
# 计算中心点坐标
(h, w) = image.shape[:2]
center = (w // 2, h // 2)
# 构造旋转矩阵
angle = 45
M = cv2.getRotationMatrix2D(center, angle, scale=1.0)
# 对图像进行旋转
rotated = cv2.warpAffine(image, M, (w, h))
# 显示旋转后的图像
cv2.imshow("Rotated Image", rotated)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
python图像批处理旋转90
在Python中,我们可以使用PIL (Python Imaging Library) 或其更新版Pillow库来进行图像的批处理,包括旋转90度的操作。下面是一个简单的示例:
```python
from PIL import Image
def rotate_90_batch(file_list):
rotated_images = []
for file in file_list:
with Image.open(file) as im:
# 图像以顺时针旋转90度
rotated = im.rotate(90)
# 保存旋转后的图片,这里假设文件名不变,实际应用中可能需要修改
rotated.save(f"rotated_{file}")
rotated_images.append(rotated)
return rotated_images
# 使用方法
image_files = ['image1.jpg', 'image2.png', 'image3.gif'] # 替换为你要旋转的实际文件路径
rotated_list = rotate_90_batch(image_files)
```
这个函数会读取指定目录下的所有图像文件,对每一幅图像旋转90度,并将结果保存下来。`rotate_90`函数会返回旋转后的Image对象,如果需要批量操作并保持原图,可以将每个旋转后的对象添加到列表中。
阅读全文
相关推荐
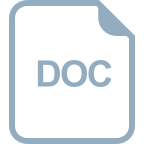
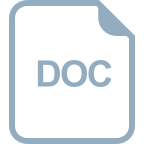
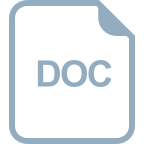
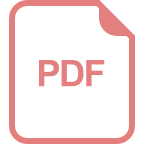
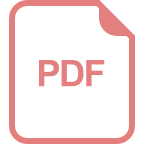
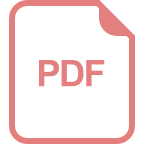
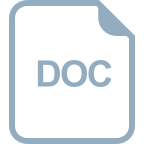
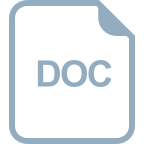
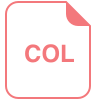
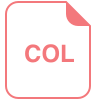
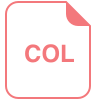
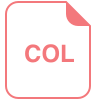
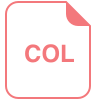



