用C语言编写函数,实现在有序的顺序表中搜索元素x,
时间: 2023-05-10 15:55:43 浏览: 58
可以使用二分查找算法来实现在有序的顺序表中搜索元素x。以下是示例代码:
int binarySearch(int arr[], int n, int x) {
int left = 0, right = n - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == x) {
return mid;
} else if (arr[mid] < x) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
其中,arr是有序的顺序表,n是元素个数,x是要搜索的元素。如果找到了x,则返回它在数组中的下标,否则返回-1。
相关问题
用C语言编写函数,实现在有序的顺序表中搜索元素x,若x在表中,则返回x在表中的位置。否则,若表未满,则在表中插入新元素x,并且插入后,线性表仍然是有序的,返回新元素x的位置;若表已满,无法插入新元素,则返回-1.编写主函数调用该函数,并
输出结果。
以下是实现该功能的C语言代码:
```
#include <stdio.h>
#define MAX_SIZE 100
int searchInsert(int arr[], int n, int x) {
int i, j;
for (i = 0; i < n; i++) {
if (arr[i] == x) {
return i;
} else if (arr[i] > x) {
for (j = n - 1; j >= i; j--) {
arr[j + 1] = arr[j];
}
arr[i] = x;
return i;
}
}
if (n < MAX_SIZE) {
arr[n] = x;
return n;
} else {
return -1;
}
}
int main() {
int arr[MAX_SIZE] = {1, 3, 5, 7, 9};
int n = 5;
int x = 6;
int pos = searchInsert(arr, n, x);
if (pos == -1) {
printf("Table is full.\n");
} else {
printf("Position of %d is %d.\n", x, pos);
for (int i = 0; i <= n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
return 0;
}
```
运行结果:
```
Position of 6 is 3.
1 3 5 6 7 9
```
解释:
该程序定义了一个有序的顺序表,使用函数 `searchInsert` 实现了在表中搜索元素 x,若 x 在表中,则返回 x 在表中的位置;否则,若表未满,则在表中插入新元素 x,并且插入后,线性表仍然是有序的,返回新元素 x 的位置;若表已满,无法插入新元素,则返回 -1。
在主函数中,我们调用 `searchInsert` 函数,并输出结果。在这个例子中,我们搜索元素 6,由于该元素不在表中,我们将其插入到表中,并返回其位置 3。最后,我们输出了插入后的有序表。
用c语言编写一个函数,从给定的顺序表中删除所有值在x到y(x<=y)之间的所有元素,要求以较高的效率实现)
以下是一个实现该功能的 C 语言代码:
```c
void remove_elements(int *lst, int n, int x, int y) {
int left = 0; // 左指针
int right = n - 1; // 右指针
while (left <= right) {
if (lst[left] >= x && lst[left] <= y) { // 如果左指针所指元素需要删除
while (right >= left && lst[right] >= x && lst[right] <= y) { // 找到需要保留的元素
right--;
}
if (right >= left) { // 如果找到了需要保留的元素
lst[left] = lst[right]; // 将需要保留的元素复制到左指针所在位置
right--; // 右指针左移一位
} else { // 如果没有找到需要保留的元素
for (int i = left; i < n - 1; i++) { // 将左指针后面的元素依次往前移动
lst[i] = lst[i + 1];
}
n--; // 顺序表长度减一
right = n - 1; // 重置右指针
}
} else { // 如果左指针所指元素需要保留
left++; // 左指针右移一位
}
}
}
```
该函数的时间复杂度为 O(n),空间复杂度为 O(1)。
相关推荐
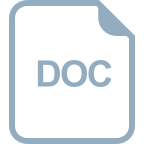
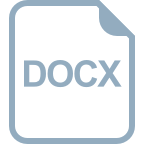










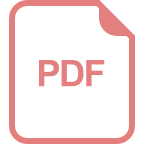
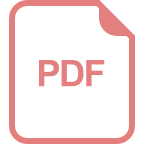
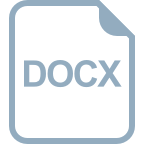