让用户输入一个月份 返回这个月的天数
时间: 2023-05-24 11:02:54 浏览: 86
A:请使用以下代码实现:
```python
month = int(input("请输入一个月份(1-12):"))
if month in [1, 3, 5, 7, 8, 10, 12]:
print("这个月有31天")
elif month in [4, 6, 9, 11]:
print("这个月有30天")
elif month == 2:
year = int(input("请输入一个年份:"))
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
print("这个月有29天")
else:
print("这个月有28天")
else:
print("输入有误,请重新输入")
```
代码的逻辑是先判断月份是否在1、3、5、7、8、10、12中,如果是,则这个月有31天;如果不是,则判断月份是否在4、6、9、11中,如果是,则这个月有30天;如果也不是,则判断月份是否为2,如果是,则需要让用户输入一个年份,再根据闰年的规则判断这个月有28天还是29天。如果月份既不在1到12中,也不是2,则输出输入有误的提示。
相关问题
让用户输入一个月份 返回这个月的天数 用break
来跳出循环并输出结果。
A:
```python
while True:
month = input("请输入月份:")
if month.isdigit() and 1 <= int(month) <= 12:
break # 输入合法,跳出循环
print("输入无效,请重新输入!")
month = int(month)
if month == 2:
print("这个月份有28天")
else:
if month in [1, 3, 5, 7, 8, 10, 12]:
print("这个月份有31天")
else:
print("这个月份有30天")
```
这里使用了`while True`循环,不断要求用户输入一个月份。如果输入无效(非数字或数字不在1-12之间),则打印提示信息并重新循环。如果输入合法,那么通过`break`跳出循环。
接下来对月份进行判断,并输出有多少天。对于2月份,直接输出28天;对于其他月份,判断它是否为31天的月份,如果是就输出31天,否则输出30天。
用户 输入一个年份 在输入一个 月份 返回 对应年份 对应月份的天数 (结合 闰年判断 处理 2月) 用if switch case**********
要根据用户输入的年份和月份来返回对应的天数,可以使用if语句或switch case语句来实现。首先需要判断年份是否为闰年,然后再根据月份返回对应的天数。
以下是使用if语句实现的示例代码:
```python
def get_days(year, month):
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0: # 判断是否为闰年
if month == 2: # 闰年的2月有29天
return 29
else:
if month == 2: # 非闰年的2月有28天
return 28
if month in [1, 3, 5, 7, 8, 10, 12]: # 大月有31天
return 31
elif month in [4, 6, 9, 11]: # 小月有30天
return 30
else:
return None # 输入的月份无效
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
days = get_days(year, month)
if days is not None:
print(f"{year}年{month}月有{days}天")
else:
print("输入的月份无效")
```
使用switch case语句实现的示例代码如下:
```python
def get_days(year, month):
is_leap_year = False
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0: # 判断是否为闰年
is_leap_year = True
days = None
if month == 2:
if is_leap_year:
days = 29
else:
days = 28
elif month in [1, 3, 5, 7, 8, 10, 12]:
days = 31
elif month in [4, 6, 9, 11]:
days = 30
return days
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
days = get_days(year, month)
if days is not None:
print(f"{year}年{month}月有{days}天")
else:
print("输入的月份无效")
```
阅读全文
相关推荐
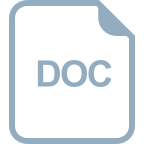
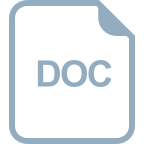
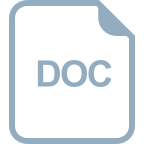









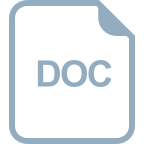
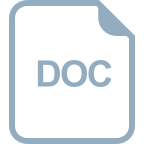
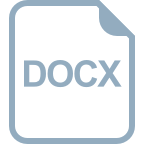