C++ string "/aa/aaa/aaa/aa.txt" 怎么将/aa/aaa/aaa/ 截取出来
时间: 2023-07-09 07:53:33 浏览: 72
你可以使用 C++ 标准库中的 `string` 类型的 `substr` 函数来截取字符串。具体操作如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string path = "/aa/aaa/aaa/aa.txt";
size_t pos = path.find_last_of('/');
string sub_path = path.substr(0, pos+1);
cout << sub_path << endl; // 输出 /aa/aaa/aaa/
return 0;
}
```
在上面的代码中,首先定义了一个 `path` 变量,表示需要截取的路径。然后使用 `find_last_of` 函数找到最后一个 `/` 的位置,并将这个位置保存在 `pos` 变量中。最后使用 `substr` 函数截取从字符串开头到 `pos+1` 的子串,即 `/aa/aaa/aaa/`。
相关问题
c/c++截取字符串string
在 C++ 中,可以使用 `substr` 函数来截取一个 `string` 类型的字符串。
`substr` 函数的语法如下:
```c++
string substr (size_t pos, size_t len) const;
```
其中,`pos` 是起始位置,`len` 是截取的长度。
例如,要截取一个 `string` 类型的字符串 `str` 的前 5 个字符,可以使用以下代码:
```c++
string str = "Hello, world!";
string result = str.substr(0, 5);
```
上述代码会将字符串 `str` 中从位置 0 开始的 5 个字符截取出来,并赋值给 `result`。
需要注意的是,`substr` 函数会返回一个新的 `string` 对象,因此原有的 `string` 对象不会受到影响。
c++ 截取字符串中//
C++中可以使用substr()函数来截取字符串,该函数的语法如下:
string substr (size_t pos, size_t len) const;
其中,pos表示要截取的子字符串的起始位置,len表示要截取的子字符串的长度。例如,以下代码截取了字符串str中从第3个字符开始的5个字符:
string str = "Hello, world!";
string sub = str.substr(2, 5); // sub为"llo, "
相关推荐
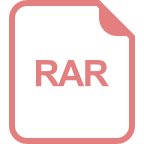
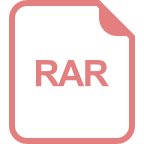
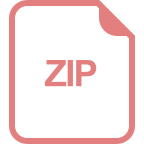












