python图像频域滤波
时间: 2023-11-06 13:58:35 浏览: 118
Python中可以使用numpy和opencv库进行图像频域滤波。
1.使用numpy库实现图像频域滤波
频域滤波的步骤是:
(1)读入图像
(2)将图像转换为灰度图
(3)进行傅里叶变换,得到频域图像
(4)设计滤波器
(5)对频域图像进行滤波操作
(6)进行傅里叶逆变换,得到滤波后的图像
代码示例:
```python
import cv2
import numpy as np
# 读入图像
img = cv2.imread('img.jpg')
# 将图像转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 进行傅里叶变换,得到频域图像
f = np.fft.fft2(gray)
fshift = np.fft.fftshift(f)
# 构建高通滤波器
rows, cols = gray.shape
crow, ccol = rows // 2, cols // 2
mask = np.ones((rows, cols), np.uint8)
mask[crow - 30: crow + 30, ccol - 30: ccol + 30] = 0
# 对频域图像进行滤波操作
fshift = fshift * mask
# 进行傅里叶逆变换,得到滤波后的图像
ishift = np.fft.ifftshift(fshift)
i = np.fft.ifft2(ishift)
result = np.abs(i)
# 显示原图和滤波后的图像
cv2.imshow('Original', gray)
cv2.imshow('Result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
2.使用opencv库实现图像频域滤波
OpenCV提供了cv2.dft()和cv2.idft()函数,可以方便地实现图像的傅里叶变换和逆变换。与numpy库相比,opencv库的实现更加简单。频域滤波的步骤与上面的步骤相同。
代码示例:
```python
import cv2
import numpy as np
# 读入图像
img = cv2.imread('img.jpg')
# 将图像转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 进行傅里叶变换,得到频域图像
dft = cv2.dft(np.float32(gray), flags=cv2.DFT_COMPLEX_OUTPUT)
dft_shift = np.fft.fftshift(dft)
# 构建高通滤波器
rows, cols = gray.shape
crow, ccol = rows // 2, cols // 2
mask = np.ones((rows, cols, 2), np.uint8)
mask[crow - 30: crow + 30, ccol - 30: ccol + 30] = 0
# 对频域图像进行滤波操作
fshift = dft_shift * mask
# 进行傅里叶逆变换,得到滤波后的图像
ishift = np.fft.ifftshift(fshift)
i = cv2.idft(ishift)
result = cv2.magnitude(i[:, :, 0], i[:, :, 1])
# 显示原图和滤波后的图像
cv2.imshow('Original', gray)
cv2.imshow('Result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐



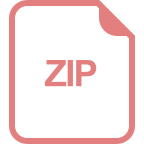
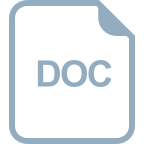
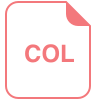






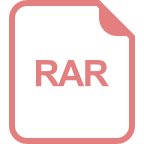
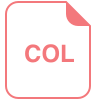
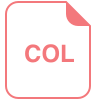
