python对图像进行频域滤波
时间: 2023-05-26 10:06:50 浏览: 183
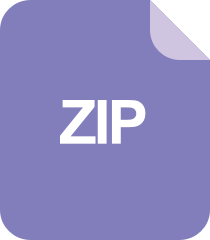
基于python的频域滤波(主要设计频域滤波器)(有丰富的图片)
Python可以使用NumPy和OpenCV库对图像进行频域滤波。
在NumPy中,可以使用FFT(快速傅里叶变换)和IFFT(逆快速傅里叶变换)函数进行频域滤波。首先,读取图像并将其转化为灰度图像:
```python
import cv2
import numpy as np
img = cv.imread('image.jpg', 0)
```
然后,计算图像的傅里叶变换:
```python
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
```
进行滤波操作,例如使用高通滤波器:
```python
rows, cols = img.shape
crow, ccol = rows/2, cols/2
# 生成高通滤波器
mask = np.ones((rows, cols), np.uint8)
mask[crow-30:crow+30, ccol-30:ccol+30] = 0
# 应用高通滤波器
fshift *= mask
# 进行逆傅里叶变换
f_ishift = np.fft.ifftshift(fshift)
img_back = np.fft.ifft2(f_ishift)
img_back = np.abs(img_back)
```
最后,将滤波后的图像保存:
```python
cv2.imwrite('filtered_image.jpg', img_back)
```
在OpenCV中,可以使用cv2.dft()和cv2.idft()函数进行傅里叶变换和逆傅里叶变换:
```python
img = cv2.imread('image.jpg', 0)
dft = cv2.dft(np.float32(img), flags=cv2.DFT_COMPLEX_OUTPUT)
dft_shift = np.fft.fftshift(dft)
# 生成高通滤波器
rows, cols = img.shape
crow, ccol = rows/2, cols/2
mask = np.ones((rows, cols, 2), np.uint8)
mask[crow-30:crow+30, ccol-30:ccol+30] = 0
# 应用高通滤波器
fshift = dft_shift*mask
f_ishift = np.fft.ifftshift(fshift)
img_back = cv2.idft(f_ishift)
img_back = cv2.magnitude(img_back[:,:,0], img_back[:,:,1])
cv2.imwrite('filtered_image.jpg', img_back)
```
以上代码只是一个示例,具体的滤波器和操作方式需要根据实际需求进行选择和调整。
阅读全文
相关推荐
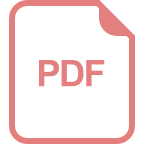
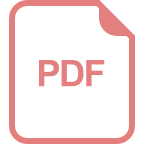
















