用c++写一个拓扑排序
时间: 2023-03-05 13:23:07 浏览: 86
拓扑排序是一种常用的图算法,可以用来解决有向无环图的排序问题。下面是用C语言实现的拓扑排序代码:
```
#include <stdio.h>
#define MAX_VERTEX 100
int edge[MAX_VERTEX][MAX_VERTEX]; // 存储图的邻接矩阵
int visited[MAX_VERTEX]; // 标记已访问的节点
int n; // 节点数量
void topologicalSort(int node, int* stack, int* top) {
visited[node] = 1;
int i;
for (i = 0; i < n; i++) {
if (edge[node][i] && !visited[i]) {
topologicalSort(i, stack, top);
}
}
stack[++(*top)] = node;
}
void topoSort() {
int stack[MAX_VERTEX], top = -1;
int i;
for (i = 0; i < n; i++) {
if (!visited[i]) {
topologicalSort(i, stack, &top);
}
}
printf("拓扑排序结果:\n");
while (top >= 0) {
printf("%d ", stack[top--]);
}
}
int main() {
// 初始化邻接矩阵
int i, j, m;
scanf("%d%d", &n, &m);
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
edge[i][j] = 0;
}
visited[i] = 0;
}
// 读入有向边
int a, b;
for (i = 0; i < m; i++) {
scanf("%d%d", &a, &b);
edge[a][b] = 1;
}
// 进行拓扑排序
topoSort();
return 0;
}
```
这段代码先通过邻接矩阵表示有向图,然后进行深度优先遍历,遍历过程中将访问过的节点压入栈中。最后依次从栈中弹出节点,得到的结果就是拓扑排序的结果。
阅读全文
相关推荐
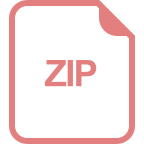
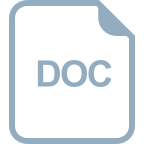
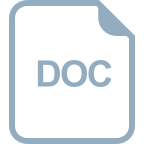
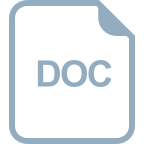
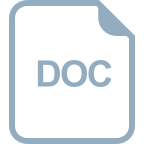
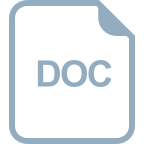









