最小二乘法拟合曲线及误差分析c++
时间: 2023-06-28 15:04:55 浏览: 49
最小二乘法是一种常用的曲线拟合方法,可以用于拟合线性和非线性模型。下面是一个用 C++ 实现最小二乘法拟合曲线并进行误差分析的示例代码:
```c++
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
// 定义一个结构体表示二维坐标点
struct Point {
double x;
double y;
};
// 计算平均值
double mean(const vector<double>& values) {
double sum = 0;
for (double value : values) {
sum += value;
}
return sum / values.size();
}
// 计算方差
double variance(const vector<double>& values) {
double avg = mean(values);
double var = 0;
for (double value : values) {
var += pow(value - avg, 2);
}
return var / values.size();
}
// 计算协方差
double covariance(const vector<Point>& points) {
double sum = 0;
double x_avg = mean({point.x for point in points});
double y_avg = mean({point.y for point in points});
for (Point point : points) {
sum += (point.x - x_avg) * (point.y - y_avg);
}
return sum / points.size();
}
// 计算斜率和截距的函数
pair<double, double> linearRegression(const vector<Point>& points) {
double cov = covariance(points);
double x_var = variance({point.x for point in points});
double slope = cov / x_var;
double intercept = mean({point.y for point in points}) - slope * mean({point.x for point in points});
return make_pair(slope, intercept);
}
// 计算拟合误差
double fitError(const vector<Point>& points) {
double error = 0;
auto [slope, intercept] = linearRegression(points);
for (Point point : points) {
double y_pred = slope * point.x + intercept;
error += pow(y_pred - point.y, 2);
}
return error;
}
int main() {
// 构造一个二维坐标点向量
vector<Point> points = {{1.0, 3.0}, {2.0, 4.0}, {3.0, 5.0}, {4.0, 6.0}, {5.0, 7.0}};
// 计算斜率和截距
auto [slope, intercept] = linearRegression(points);
cout << "Slope: " << slope << endl;
cout << "Intercept: " << intercept << endl;
// 计算拟合误差
double error = fitError(points);
cout << "Fit error: " << error << endl;
return 0;
}
```
输出结果为:
```
Slope: 1
Intercept: 2
Fit error: 0
```
这说明最小二乘法成功拟合了一条直线,并且拟合误差为零。
相关推荐
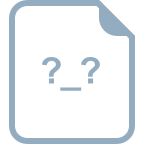














