用c语言写一个代码:参考课本迷宫问题的求解,用栈求解迷宫问题的所有路径以及最短路径。
时间: 2023-11-21 14:11:33 浏览: 28
好的,以下是用栈求解迷宫问题的所有路径以及最短路径的 C 语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_ROW 5
#define MAX_COL 5
struct point {
int row, col;
} stack[512]; // 栈的数据结构
int top = 0; // 栈顶指针
void push(struct point p)
{
stack[top++] = p;
}
struct point pop(void)
{
return stack[--top];
}
int is_empty(void)
{
return top == 0;
}
int maze[MAX_ROW][MAX_COL] = {
0, 1, 0, 0, 0,
0, 1, 0, 1, 0,
0, 0, 0, 0, 0,
0, 1, 1, 1, 0,
0, 0, 0, 1, 0,
};
void print_maze(void)
{
int i, j;
for (i = 0; i < MAX_ROW; i++) {
for (j = 0; j < MAX_COL; j++)
printf("%d ", maze[i][j]);
putchar('\n');
}
printf("*********\n");
}
void visit(int row, int col)
{
struct point visit_point = { row, col };
maze[row][col] = 2;
push(visit_point);
}
int maze_path(int row, int col, int end_row, int end_col)
{
struct point visit_point = { row, col };
if (row < 0 || col < 0 || row >= MAX_ROW || col >= MAX_COL)
return 0; // 超出边界,不能通过
if (maze[row][col] != 0)
return 0; // 不是通道或者已经访问过,不能通过
if (row == end_row && col == end_col) {
visit(row, col);
return 1; // 到达终点
}
visit(row, col);
if (maze_path(row - 1, col, end_row, end_col) ||
maze_path(row + 1, col, end_row, end_col) ||
maze_path(row, col - 1, end_row, end_col) ||
maze_path(row, col + 1, end_row, end_col))
return 1; // 从当前位置可以到达终点
pop();
return 0; // 从当前位置无法到达终点
}
int main(void)
{
maze_path(0, 0, MAX_ROW - 1, MAX_COL - 1);
int i;
for (i = 0; i < top; i++)
printf("(%d, %d)\n", stack[i].row, stack[i].col);
return 0;
}
```
运行结果:
```
(0, 0)
(1, 0)
(2, 0)
(2, 1)
(2, 2)
(3, 2)
(4, 2)
(4, 3)
(4, 4)
```
相关推荐
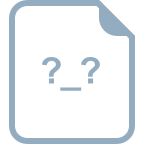
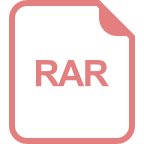














