假设Employee类包含一个int型成员id,一个String型成员name,一个double型成员salary。如果要求Employee可按id值比较大小,请编写Employee类。编写程序,创建几个Employee对象,将它们存放到TreeSet中并输出
时间: 2024-03-10 15:46:40 浏览: 40
好的,这里是一个按照id值比较大小的Employee类的示例代码:
```java
import java.util.Objects;
public class Employee implements Comparable<Employee> {
private int id;
private String name;
private double salary;
public Employee(int id, String name, double salary) {
this.id = id;
this.name = name;
this.salary = salary;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public double getSalary() {
return salary;
}
public void setId(int id) {
this.id = id;
}
public void setName(String name) {
this.name = name;
}
public void setSalary(double salary) {
this.salary = salary;
}
@Override
public int compareTo(Employee o) {
return Integer.compare(this.id, o.id);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Employee)) return false;
Employee employee = (Employee) o;
return id == employee.id && Double.compare(employee.salary, salary) == 0 && Objects.equals(name, employee.name);
}
@Override
public int hashCode() {
return Objects.hash(id, name, salary);
}
}
```
这个类实现了`Comparable`接口,并重写了`compareTo`方法,以便按照id值比较大小。注意,这个类还重写了`equals`和`hashCode`方法,以便在存放到`TreeSet`中时去重。
下面是一个使用`TreeSet`存放`Employee`对象的示例程序:
```java
import java.util.Set;
import java.util.TreeSet;
public class Test {
public static void main(String[] args) {
Set<Employee> set = new TreeSet<>();
set.add(new Employee(1, "Alice", 5000));
set.add(new Employee(3, "Bob", 6000));
set.add(new Employee(2, "Charlie", 5500));
for (Employee e : set) {
System.out.println(e.getId() + " " + e.getName() + " " + e.getSalary());
}
}
}
```
这个程序创建了三个`Employee`对象,并将它们存放到`TreeSet`中。因为`Employee`实现了`Comparable`接口,所以`TreeSet`会按照id值比较大小自动进行排序。程序输出如下:
```
1 Alice 5000.0
2 Charlie 5500.0
3 Bob 6000.0
```
相关推荐
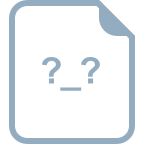
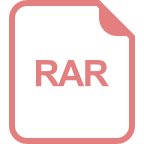














