我在用librdkafka的C API消费数据。使用 rd_kafka_subscribe 可以一次性的提交好几个topic,这时该如何进行 seek呢?
时间: 2023-05-11 14:04:01 浏览: 70
您可以使用 rd_kafka_seek 函数来进行 seek 操作。该函数可以接收一个 topic 和一个 offset,用于将消费者的偏移量设置为指定的值。例如,如果您想将消费者的偏移量设置为某个 topic 的第 10 条消息,可以使用以下代码:
rd_kafka_seek(rk, topic, 10, 5000);
其中,rk 是 rd_kafka_t 类型的指针,topic 是 rd_kafka_topic_t 类型的指针,10 是要设置的偏移量,5000 是超时时间(单位为毫秒),表示等待 seek 操作完成的最长时间。如果在超时时间内 seek 操作没有完成,函数将返回错误。
相关问题
已知一个kafka专题名字是mytopic。请使用librdkafka写一个消费者,并且每次启动时,都从同一个偏移1000开始消费。请使用rd_kafka_seek_partitions函数,并遵循librdkafka的C API
非常感谢您的问题。以下是使用librdkafka编写消费者的代码示例,该消费者从同一个偏移1000开始消费:
```
#include <librdkafka/rdkafka.h>
int main() {
rd_kafka_t *rk;
rd_kafka_conf_t *conf;
rd_kafka_topic_partition_list_t *topics;
rd_kafka_resp_err_t err;
char errstr[512];
// 创建配置对象
conf = rd_kafka_conf_new();
// 设置消费者组ID
rd_kafka_conf_set(conf, "group.id", "mygroup", errstr, sizeof(errstr));
// 创建kafka实例
rk = rd_kafka_new(RD_KAFKA_CONSUMER, conf, errstr, sizeof(errstr));
if (!rk) {
fprintf(stderr, "Failed to create new consumer: %s\n", errstr);
return 1;
}
// 添加要消费的主题
topics = rd_kafka_topic_partition_list_new(1);
rd_kafka_topic_partition_list_add(topics, "mytopic", RD_KAFKA_PARTITION_UA);
// 设置偏移量
rd_kafka_topic_partition_list_set_offset(topics, 0, 1000);
// 启动消费者
err = rd_kafka_subscribe(rk, topics);
if (err) {
fprintf(stderr, "Failed to start consuming: %s\n", rd_kafka_err2str(err));
return 1;
}
// 消费消息
while (1) {
rd_kafka_message_t *msg;
msg = rd_kafka_consumer_poll(rk, 1000);
if (!msg) {
continue;
}
if (msg->err) {
if (msg->err == RD_KAFKA_RESP_ERR__PARTITION_EOF) {
printf("Reached end of partition\n");
} else {
fprintf(stderr, "Failed to consume message: %s\n", rd_kafka_message_errstr(msg));
}
} else {
printf("Received message: %.*s\n", (int)msg->len, (char *)msg->payload);
}
rd_kafka_message_destroy(msg);
}
// 停止消费者
rd_kafka_unsubscribe(rk);
rd_kafka_topic_partition_list_destroy(topics);
rd_kafka_destroy(rk);
return 0;
}
```
请注意,此代码示例仅供参考,您需要根据实际情况进行修改和调整。
kafka消费者消费多个topic消息代码实现
可以使用 Kafka Consumer API 来消费多个 topic 的消息。具体实现代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <librdkafka/rdkafka.h>
int main(int argc, char **argv) {
rd_kafka_t *rk; /* Kafka producer instance handle */
rd_kafka_conf_t *conf; /* Temporary configuration object */
char errstr[512]; /* librdkafka API error reporting buffer */
char *brokers; /* Kafka broker(s) */
char *topics; /* Topic list to consume from */
rd_kafka_topic_partition_list_t *topic_list; /* List of topics to subscribe to */
rd_kafka_resp_err_t err; /* librdkafka API error code */
/* Check arguments */
if (argc != 3) {
fprintf(stderr, "Usage: %s <broker> <topic1,topic2,...>\n", argv[]);
exit(1);
}
brokers = argv[1];
topics = argv[2];
/* Create Kafka client configuration place-holder */
conf = rd_kafka_conf_new();
/* Set bootstrap broker(s) as a comma-separated list of
* host or host:port (default port is 9092).
* librdkafka will use the bootstrap brokers to acquire the full
* set of brokers from the cluster. */
if (rd_kafka_conf_set(conf, "bootstrap.servers", brokers, errstr, sizeof(errstr)) != RD_KAFKA_CONF_OK) {
fprintf(stderr, "%s\n", errstr);
exit(1);
}
/* Create Kafka producer instance */
rk = rd_kafka_new(RD_KAFKA_CONSUMER, conf, errstr, sizeof(errstr));
if (!rk) {
fprintf(stderr, "Failed to create Kafka producer: %s\n", errstr);
exit(1);
}
/* Create topic list */
topic_list = rd_kafka_topic_partition_list_new(1);
if (!topic_list) {
fprintf(stderr, "Failed to create topic list\n");
exit(1);
}
/* Parse topic list */
if (rd_kafka_topic_partition_list_add(topic_list, topics, RD_KAFKA_PARTITION_UA) != topic_list->cnt) {
fprintf(stderr, "Failed to parse topic list: %s\n", rd_kafka_err2str(rd_kafka_last_error()));
exit(1);
}
/* Subscribe to topic list */
err = rd_kafka_subscribe(rk, topic_list);
if (err) {
fprintf(stderr, "Failed to subscribe to topic list: %s\n", rd_kafka_err2str(err));
exit(1);
}
/* Consume messages */
while (1) {
rd_kafka_message_t *msg;
/* Poll for new messages */
msg = rd_kafka_consumer_poll(rk, 100);
if (!msg) {
continue;
}
/* Print message */
printf("Received message on topic %s (partition %d) at offset %ld:\n",
rd_kafka_topic_name(msg->rkt), msg->partition, msg->offset);
printf("%.*s\n", (int)msg->len, (char *)msg->payload);
/* Free message */
rd_kafka_message_destroy(msg);
}
/* Destroy topic list */
rd_kafka_topic_partition_list_destroy(topic_list);
/* Destroy Kafka producer instance */
rd_kafka_destroy(rk);
return ;
}
```
以上代码实现了消费多个 topic 的消息,具体实现过程如下:
1. 创建 Kafka client configuration place-holder。
2. 设置 bootstrap broker(s)。
3. 创建 Kafka producer instance。
4. 创建 topic list。
5. 解析 topic list。
6. 订阅 topic list。
7. 消费消息。
8. 销毁 topic list 和 Kafka producer instance。
注意:以上代码仅供参考,实际使用时需要根据具体情况进行修改。
相关推荐
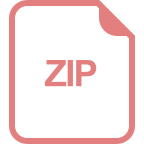
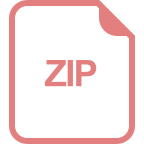
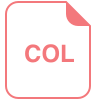
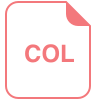
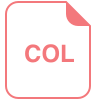
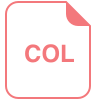
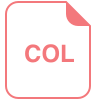







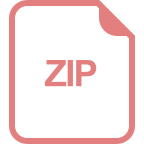