C++函数n x n 方阵里填入1,2,···,n x n,要求填成蛇形。例如,n = 4 时方阵为: 10 11 12 1 9 16 13 2 8 15 14 3 7 6 5 4
时间: 2024-12-24 19:36:10 浏览: 6
在C++中,创建一个n x n的蛇形填充矩阵可以采用递归或迭代的方式来实现。下面是一个简单的递归方法示例:
```cpp
#include <vector>
std::vector<std::vector<int>> snake_matrix(int n) {
if (n <= 0) return {};
std::vector<std::vector<int>> mat(n, std::vector<int>(n));
int start = 1, direction = 0; // start at (0, 0), move right initially
for (int i = 0; i < n * n; ++i) {
mat[start / n][start % n] = i + 1;
// Change direction after visiting the middle row or column
if ((start / n == (n - 1) / 2 && start % n != (n - 1) % 2) ||
(start % n == (n - 1) % 2 && start / n != (n - 1) / 2)) {
direction = 1 - direction;
}
// Update starting position based on direction
start += direction;
}
return mat;
}
// 示例
int main() {
int n = 4;
auto result = snake_matrix(n);
for (const auto &row : result) {
for (int num : row) {
std::cout << num << " ";
}
std::cout << "\n";
}
return 0;
}
```
在这个代码中,`snake_matrix` 函数接收一个整数 n,然后生成一个大小为 n x n 的矩阵,按照蛇形规律填充数字。当到达中间行或列时,方向会发生改变,从右上到左下或反之。
阅读全文
相关推荐
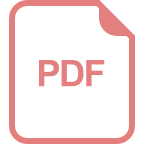
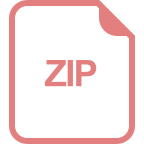
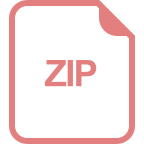
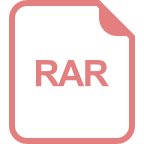
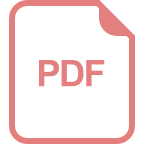
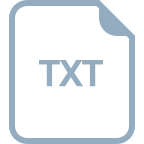
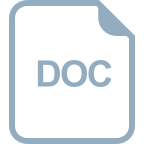
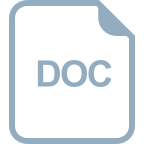
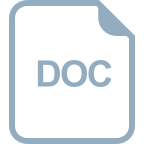
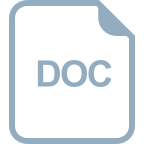
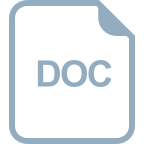





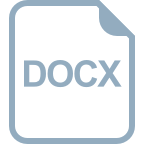
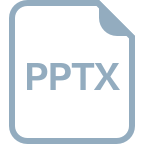
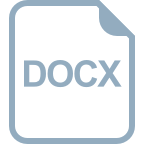