结合emit函数,说出在子组件ToDoFooter.vue中,当点击“all、active、completed”不同按钮的时候,子组件ToDoFooter.vue是怎样发送事件和数据给父组件app.vue的
时间: 2024-10-19 22:07:04 浏览: 35
在Vue中,子组件可以通过`emit`函数向其父组件发送自定义事件。假设你在`ToDoFooter.vue`组件中有三个按钮对应不同的状态(所有任务、活跃任务和已完成任务),你可以这样做:
```vue
<template>
<footer>
<button @click="handleAll">全部</button>
<button @click="handleActive">活跃</button>
<button @click="handleCompleted">已完成</button>
</footer>
</template>
<script>
import { defineComponent, emit } from 'vue';
export default defineComponent({
methods: {
handleAll() {
this.$emit('filter-todo', 'all');
},
handleActive() {
this.$emit('filter-todo', 'active');
},
handleCompleted() {
this.$emit('filter-todo', 'completed');
},
},
});
</script>
```
在这个例子中,每个按钮的`@click`事件触发相应的处理函数(如`handleAll`, `handleActive`, 或`handleCompleted`),并在这些函数内部,使用`$emit`方法发送名为`filter-todo`的自定义事件,并带上状态参数('all', 'active', 或'completed')。这表示当用户点击按钮时,`filter-todo`事件会被触发,并附带相应状态。
在`app.vue`的父组件中,你需要监听这个事件并接收并处理这个状态:
```vue
<template>
<div>
<ToDoFooter />
<!-- 根据接收到的状态更新显示内容 -->
<ul v-if="$route.query.filter === 'all'">
<!-- 显示所有任务 -->
</ul>
<ul v-if="$route.query.filter === 'active'">
<!-- 显示活跃任务 -->
</ul>
<ul v-if="$route.query.filter === 'completed'">
<!-- 显示已完成任务 -->
</ul>
</div>
</template>
<script>
import ToDoFooter from '@/components/ToDoFooter.vue';
export default {
components: {
ToDoFooter,
},
mounted() {
this.fetchTodos(); // 假设你有 fetchTodos 方法来获取初始数据
},
watch: {
'$route.query.filter': 'updateTodoList', // 当 filter 变化时调用这个方法
},
methods: {
updateTodoList(newFilter) {
// 调用 API 或者本地存储获取新的过滤后的数据
this.todos = fetchDataBasedOnFilter(newFilter);
// 更新路由查询参数以便于前端缓存
this.$router.replace({ query: { filter: newFilter } });
},
},
};
</script>
```
在这里,父组件通过`watch`监听`$route.query.filter`的变化,当从子组件接收到`filter-todo`事件时,会调用`updateTodoList`方法来更新显示的内容。
阅读全文
相关推荐
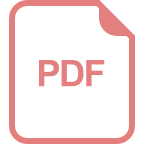
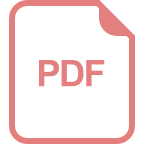
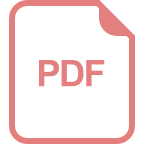















