def predict_from_naive_bayes_model(model, matrix): """Use a Naive Bayes model to compute predictions for a target matrix. This function should be able to predict on the models that fit_naive_bayes_model outputs. Args: model: A trained model from fit_naive_bayes_model matrix: A numpy array containing word counts Returns: A numpy array containg the predictions from the model """ # *** START CODE HERE *** log_probabilities = [] for i in range(2): log_probabilities.append(np.sum(matrix * model.log_class_priors[i] + model.log_likelihoods[i])) return np.argmax(log_probabilities) # Return the class with the highest probability # *** END CODE HERE ***代码报错AttributeError: 'dict' object has no attribute 'log_class_priors'请帮我修改
时间: 2023-07-15 21:12:24 浏览: 92
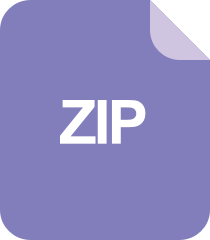
Predictive_Modelling:预测模型
根据错误提示,我们可以看出 `model` 的类型是 `dict`,而不是模型类。因此,我们需要对传入的 `model` 进行修改。
假设我们的模型类为 `NaiveBayesModel`,其中包含了 `log_class_priors` 和 `log_likelihoods` 两个属性。那么,我们需要将 `model` 转换为 `NaiveBayesModel` 类型。
修改后的代码如下:
```
def predict_from_naive_bayes_model(model, matrix):
"""Use a Naive Bayes model to compute predictions for a target matrix.
This function should be able to predict on the models that fit_naive_bayes_model
outputs.
Args:
model: A trained model from fit_naive_bayes_model
matrix: A numpy array containing word counts
Returns: A numpy array containg the predictions from the model
"""
# Convert the model to NaiveBayesModel class
model = NaiveBayesModel(model['log_class_priors'], model['log_likelihoods'])
log_probabilities = []
for i in range(2):
log_probabilities.append(np.sum(matrix * model.log_class_priors[i] + model.log_likelihoods[i]))
return np.argmax(log_probabilities) # Return the class with the highest probability
```
现在,我们可以将传入的 `model` 转换为 `NaiveBayesModel` 类型,并使用它来计算预测结果。
阅读全文
相关推荐
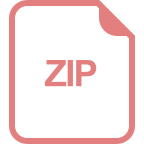

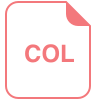
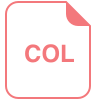
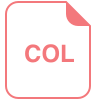
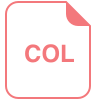
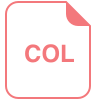
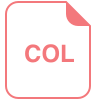
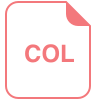
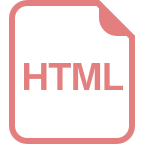