python给定一个二叉搜索树将其转换为一个已排序的双向链表,基本要求 输入数据是一组数据元素来构建一个二叉搜索树,您应该设计 算法实现转换过程,并验证代码的准确性 多个测试数据。 该输出内容包括以下内容: (1)打印二叉搜索树 (2)遍历二叉搜索树 (3)打印转换后的双链表 (4)以正的顺序和反向的顺序输出双链表中的所有元素
时间: 2024-02-12 22:03:40 浏览: 68
好的,这是一个比较具体的问题,我可以给出一个可能的解法。
首先我们需要定义一个二叉搜索树的节点类:
```python
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
```
接下来我们可以编写一个函数来构建二叉搜索树。这个函数需要接受一个列表作为输入,然后将列表中的元素插入到二叉搜索树中:
```python
def build_bst(nums):
if not nums:
return None
root = Node(nums[0])
for num in nums[1:]:
insert(root, num)
return root
def insert(root, value):
if root is None:
return Node(value)
if value < root.value:
root.left = insert(root.left, value)
else:
root.right = insert(root.right, value)
return root
```
接下来我们需要将这个二叉搜索树转换成双向链表。我们可以定义一个函数来进行中序遍历,并在遍历的过程中进行节点之间的连接:
```python
def bst_to_dll(root):
if root is None:
return None
head, tail = inorder_traversal(root)
return head
def inorder_traversal(root):
if root is None:
return None, None
left_head, left_tail = inorder_traversal(root.left)
right_head, right_tail = inorder_traversal(root.right)
node = Node(root.value)
if left_head is not None:
left_tail.right = node
node.left = left_tail
else:
left_head = node
if right_head is not None:
right_head.left = node
node.right = right_head
else:
right_tail = node
return left_head, right_tail
```
最后我们可以编写一个函数来进行测试。这个函数需要构建一个二叉搜索树,然后将它转换成双向链表,并分别打印二叉搜索树、遍历结果和双向链表:
```python
def test():
nums = [5, 3, 7, 2, 4, 6, 8]
root = build_bst(nums)
print("Binary Search Tree:")
print_tree(root)
print("Inorder Traversal:")
print_list(inorder_traversal(root)[0])
print("Doubly Linked List (Forward):")
head = bst_to_dll(root)
while head is not None:
print(head.value, end=" ")
head = head.right
print()
print("Doubly Linked List (Backward):")
tail = inorder_traversal(root)[1]
while tail is not None:
print(tail.value, end=" ")
tail = tail.left
print()
def print_tree(root):
if root is None:
return
print(root.value)
print_tree(root.left)
print_tree(root.right)
def print_list(head):
while head is not None:
print(head.value, end=" ")
head = head.right
print()
```
这样,我们就可以通过 `test()` 函数来进行测试了。注意,这个算法的时间复杂度是 $O(n)$,其中 $n$ 是二叉搜索树中节点的个数。
阅读全文
相关推荐
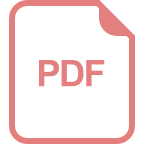
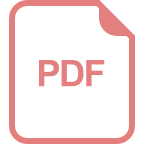
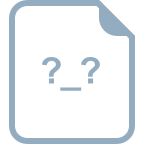





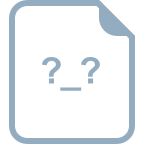
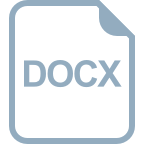
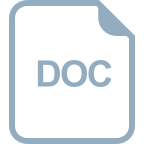
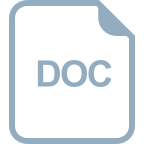
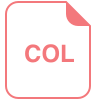
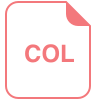
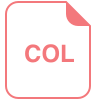
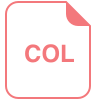
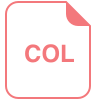
