在空的Dataframe里添加数据
时间: 2024-02-21 16:58:36 浏览: 133
可以使用pandas库中的append()方法向空的Dataframe中添加数据。具体操作如下:
1. 创建一个空的Dataframe:
```python
import pandas as pd
df = pd.DataFrame(columns=['列名1', '列名2', ...])
```
2. 使用append()方法向Dataframe中添加数据:
```python
df = df.append({'列名1': 值1, '列名2': 值2, ...}, ignore_index=True)
```
其中,ignore_index=True参数表示忽略原有的索引,重新生成索引。
3. 重复上述步骤,添加更多的数据。
注意:在使用append()方法添加数据时,需要保证每个数据字典中的键名和Dataframe的列名一一对应,否则会出现数据错位的情况。
相关问题
python 空dataframe中添加数据
可以通过以下方式向空的dataframe中添加数据:
```
import pandas as pd
df = pd.DataFrame() # 空的dataframe
new_data = {'col1': 1, 'col2': 2} # 新数据
df = df.append(new_data, ignore_index=True) # 向dataframe中添加新数据
```
其中,new_data是一个字典,包含了新添加的数据,ignore_index参数用于重新为新行编号。
对空白dataframe添加数据
要向空白的DataFrame添加数据,可以使用以下方法:
1. 使用字典创建DataFrame并添加数据
```python
import pandas as pd
# 创建空白DataFrame
df = pd.DataFrame(columns=['name', 'age', 'gender'])
# 添加数据
df = df.append({'name': 'Alice', 'age': 25, 'gender': 'F'}, ignore_index=True)
df = df.append({'name': 'Bob', 'age': 30, 'gender': 'M'}, ignore_index=True)
```
2. 使用列表创建DataFrame并添加数据
```python
import pandas as pd
# 创建空白DataFrame
df = pd.DataFrame(columns=['name', 'age', 'gender'])
# 添加数据
df.loc[len(df)] = ['Alice', 25, 'F']
df.loc[len(df)] = ['Bob', 30, 'M']
```
无论哪种方法,都需要指定DataFrame的列名,并使用`append`或`loc`方法添加数据。在使用`loc`方法时,需要指定要添加的行的索引。可以使用`len(df)`来获取当前DataFrame的行数,从而确定新行的索引。
阅读全文
相关推荐
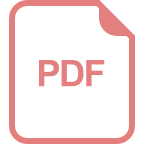














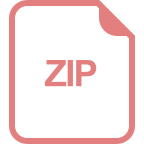