用java语言编一个程序。用对象模拟实现一个售货过程。
时间: 2023-06-16 12:08:22 浏览: 49
好的,下面是一个简单的 Java 程序,用对象模拟实现一个售货过程。
```java
import java.util.Scanner;
class Product {
private String name;
private double price;
private int quantity;
public Product(String name, double price, int quantity) {
this.name = name;
this.price = price;
this.quantity = quantity;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public double calculatePrice(int quantity) {
return price * quantity;
}
}
class Cart {
private Product[] products;
private int index;
public Cart(int size) {
products = new Product[size];
index = 0;
}
public void addProduct(Product product) {
if (index < products.length) {
products[index++] = product;
System.out.println("添加商品成功!");
} else {
System.out.println("购物车已满!");
}
}
public double calculateTotalPrice() {
double totalPrice = 0;
for (int i = 0; i < index; i++) {
totalPrice += products[i].getPrice() * products[i].getQuantity();
}
return totalPrice;
}
public void printProducts() {
System.out.println("购物车中的商品:");
for (int i = 0; i < index; i++) {
System.out.println(products[i].getName() + " 价格:" + products[i].getPrice() + " 数量:" + products[i].getQuantity());
}
}
public void checkout() {
double totalPrice = calculateTotalPrice();
System.out.println("总计:" + totalPrice);
System.out.println("请输入支付金额:");
Scanner scanner = new Scanner(System.in);
double payment = scanner.nextDouble();
if (payment >= totalPrice) {
System.out.println("找零:" + (payment - totalPrice));
} else {
System.out.println("支付金额不足!");
}
}
}
public class ShoppingCart {
public static void main(String[] args) {
Product[] products = new Product[3];
products[0] = new Product("可乐", 3.0, 10);
products[1] = new Product("薯片", 5.0, 5);
products[2] = new Product("巧克力", 10.0, 3);
Cart cart = new Cart(5);
cart.addProduct(products[0]);
cart.addProduct(products[1]);
cart.addProduct(products[2]);
cart.printProducts();
cart.checkout();
}
}
```
程序中定义了两个类,`Product` 和 `Cart`。`Product` 表示一种商品,包括商品名称、价格和数量;`Cart` 表示购物车,包括商品列表、添加商品、计算总价、打印商品和结算等方法。
在 `main` 方法中,我们创建了三种商品,然后将它们添加到购物车中,最后打印商品列表并结算。如果支付金额足够,程序会输出找零金额;否则会提示支付金额不足。
你可以根据自己的需求修改程序,例如添加删除商品、修改商品数量等。
相关推荐
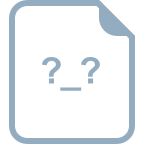
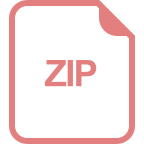














