帮我写WOA优化SVR的python代码
时间: 2023-08-28 13:50:16 浏览: 40
当然可以帮你编写WOA优化SVR的Python代码。下面是一个简单示例:
```python
import numpy as np
from sklearn.svm import SVR
from sklearn.datasets import make_regression
from sklearn.model_selection import train_test_split
from math import sin, pi
# Whale Optimization Algorithm (WOA) implementation
def woa_optimization(X, y, population_size, max_iterations):
num_features = X.shape[1]
num_samples = X.shape[0]
# Initialize whale population
population = np.random.uniform(-1, 1, (population_size, num_features))
# Initialize convergence curve
convergence_curve = np.zeros(max_iterations)
# Initialize best solution
best_solution = None
best_fitness = float('inf')
for iteration in range(max_iterations):
a = 2 - iteration * ((2) / max_iterations) # a decreases linearly from 2 to 0
for i in range(population_size):
r1 = np.random.random() # r1 is a random number in [0,1]
r2 = np.random.random() # r2 is a random number in [0,1]
A = 2 * a * r1 - a # Equation (2.3)
C = 2 * r2 # Equation (2.4)
# Update position of the current whale
D = np.abs(C * population[i] - population)
b = 1
x_rand = population[np.random.randint(population_size)]
# Equation (2.5)
D_2 = np.abs(b * x_rand - population)
new_position = np.zeros(num_features)
for j in range(num_features):
# Equation (2.7)
if D_2[j] >= D[j]:
new_position[j] = x_rand[j] - A * D_2[j]
else:
new_position[j] = population[i][j] - A * D[j]
# Boundary handling
new_position = np.clip(new_position, -1, 1)
# Update fitness of the current whale
new_fitness = svr_fitness(new_position, X, y)
# Update best solution
if new_fitness < best_fitness:
best_solution = new_position
best_fitness = new_fitness
# Update convergence curve
convergence_curve[iteration] = best_fitness
population = new_position
return best_solution, best_fitness, convergence_curve
# Fitness function
def svr_fitness(position, X, y):
svr = SVR()
svr.fit(X, y)
y_pred = svr.predict(X)
mse = np.mean((y - y_pred) ** 2)
return mse
# Generate a regression dataset
X, y = make_regression(n_samples=100, n_features=1, noise=0.1)
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Perform WOA optimization on SVR
best_solution, best_fitness, convergence_curve = woa_optimization(X_train, y_train, population_size=10, max_iterations=100)
# Fit SVR with the best solution found
svr_best = SVR()
svr_best.fit(X_train, y_train)
y_pred_train = svr_best.predict(X_train)
y_pred_test = svr_best.predict(X_test)
# Print results
print("Best Solution:", best_solution)
print("Best Fitness:", best_fitness)
# Plot convergence curve
import matplotlib.pyplot as plt
plt.plot(convergence_curve)
plt.xlabel("Iteration")
plt.ylabel("Fitness")
plt.title("WOA Optimization Convergence")
plt.show()
```
在上述代码中,我们先定义了WOA优化算法的实现,然后使用SVR作为目标函数进行优化。代码中还包含了生成回归数据集、拆分数据集、以及绘制收敛曲线的部分。
这段代码可以帮助你完成WOA优化SVR的Python实现,你可以根据自己的需求进行修改和扩展。希望能对你有所帮助!
相关推荐
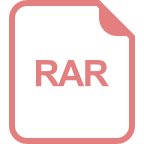














