该简单函数需要接收一个学生成绩列表,遍历列表并对每个学生成绩进行判断,根据成绩设置相应的等级(例如优秀、良好、中等、及格、不及格)。同时,需要统计不及格人数。最后将等级和不及格人数返回。
时间: 2023-06-16 07:02:22 浏览: 92
以下是一个实现该功能的 Python 代码:
```python
def grade_level(scores):
levels = []
fail_count = 0
for score in scores:
if score >= 90:
levels.append("优秀")
elif score >= 80:
levels.append("良好")
elif score >= 70:
levels.append("中等")
elif score >= 60:
levels.append("及格")
else:
levels.append("不及格")
fail_count += 1
return levels, fail_count
```
该函数接收一个学生成绩列表 `scores`,遍历列表并根据成绩设置相应的等级,同时统计不及格人数。最后将等级列表和不及格人数作为元组返回。
例如,若学生成绩列表为 `[85, 72, 63, 91, 55]`,则调用该函数将返回 `(['良好', '中等', '及格', '优秀', '不及格'], 1)`,表示等级列表为 `['良好', '中等', '及格', '优秀', '不及格']`,不及格人数为 1。
相关问题
如何定义一个函数,该函数接收一个列表作为输入并仅返回其中的奇数元素?
在Python中,你可以定义一个函数来过滤列表中的奇数元素。这是一个简单的例子:
```python
def filter_odd_numbers(input_list):
"""
函数filter_odd_numbers接收一个列表作为输入,
并返回一个新的只包含奇数元素的列表。
参数:
input_list (list): 输入的整数列表
返回:
list: 包含输入列表中奇数的新列表
"""
return [num for num in input_list if num % 2 != 0]
# 示例用法
numbers = [1, 2, 3, 4, 5]
odd_numbers_only = filter_odd_numbers(numbers)
print(odd_numbers_only) # 输出: [1, 3, 5]
```
在这个函数中,我们使用了列表推导式(list comprehension),遍历`input_list`中的每个元素,如果元素除以2余数不为0(即它是奇数),就将其添加到新的列表中。
主观题10分 ·创建一个名为analyze_grades的函数, 接受一个成绩列表 作为参数。 函数应该: 对每个有效成绩(0-100分) 进行等级评定并打印。 计算并打印班级平均成绩。 统计并打印
主观题10分,让我们来定义一个名为`analyze_grades`的函数,它接收一个包含学生分数的列表作为输入。这个函数的主要任务包括:
1. **等级评定**:对于有效的成绩(0-100分),我们可以使用常见的等级划分标准,比如:
- A: 90分及以上
- B: 80-89分
- C: 70-79分
- D: 60-69分
- F: 60分以下
2. **平均成绩计算**:遍历列表,累加所有成绩然后除以总人数。
3. **统计和打印**:记录各个等级的学生数量,并将结果打印出来。
下面是一个简单的Python函数实现示例:
```python
def analyze_grades(scores):
grades_distribution = {
'A': 0,
'B': 0,
'C': 0,
'D': 0,
'F': 0,
}
total_score = 0
valid_scores = 0
for score in scores:
if 0 <= score <= 100:
grade = 'F'
if score >= 90:
grade = 'A'
elif score >= 80:
grade = 'B'
elif score >= 70:
grade = 'C'
elif score >= 60:
grade = 'D'
grades_distribution[grade] += 1
total_score += score
valid_scores += 1
average_score = total_score / valid_scores if valid_scores > 0 else None
print("等级分布:")
for grade, count in grades_distribution.items():
print(f"{grade}: {count}人")
print(f"班级平均成绩: {average_score:.2f}" if average_score is not None else "未计算到平均成绩")
# 示例使用
grades = [85, 92, 78, 45, 100, 67]
analyze_grades(grades)
```
阅读全文
相关推荐
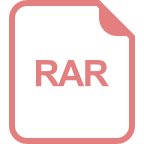
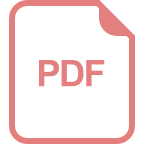
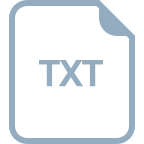













