如何使用C++和PCL(Point Cloud Library)来处理一个有序点集并确定每个点周围的按照特定方向排列的邻接点?
时间: 2024-12-23 17:22:12 浏览: 5
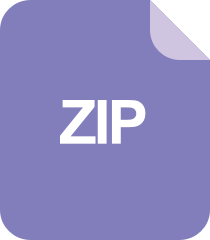
基于C++和PCL库等实现了点云处理算法+源码(高分优秀项目)
在C++中使用PCL(Point Cloud Library)处理有序点集,并确定每个点周围按照特定方向排列的邻接点,通常需要以下几个步骤:
1. **包含头文件**:首先,你需要在代码中包含PCL相关的库头文件,例如`pcl/io/pcd_io.h`、`pcl/features/normal_3d.h`等。
```cpp
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
#include <pcl/features/normal_3d.h>
```
2. **读取点云数据**:使用`pcl::io::loadPCDFile()`函数加载点云数据到`pcl::PointCloud<pcl::PointXYZ>`或者其他适合的点类型。
```cpp
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
if (pcl::io::loadPCDFile<pcl::PointXYZ>("input.pcd", *cloud) == -1)
{
// 处理错误
}
```
3. **计算邻接点**:你可以使用`pcl::search::KdTree`来查找每个点的邻居。比如找到半径范围内的点,然后按特定方向排序,如顺时针或逆时针。
```cpp
pcl::search::KdTree<pcl::PointXYZ> tree;
tree.setInputCloud(cloud);
std::vector<int> indices;
std::vector<float> distances;
int k = 5; // 指定搜索k个最近邻点
tree.searchKnn(point_index, k, indices, distances);
// 对indices数组进行排序,这里假设你要按x轴正向排序
std::sort(indices.begin(), indices.end(),
[point_index](const int& i1, const int& i2) {
return cloud->points[i1].x > cloud->points[i2].x;
});
```
4. **处理结果**:现在`indices`包含了按照指定方向排序的邻接点索引。你可以遍历这个列表来访问这些邻接点,并对它们进行进一步的操作,如提取特征、计算表面法线等。
```cpp
for (size_t i = 0; i < indices.size(); ++i)
{
pcl::PointXYZ neighbor = cloud->points[indices[i]];
// ... 进行后续操作
}
```
阅读全文
相关推荐
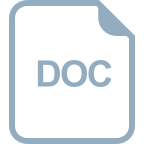
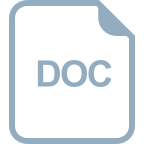
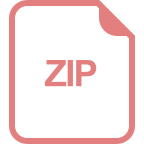
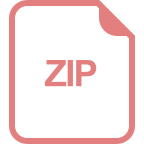

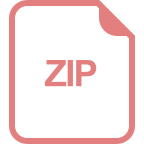
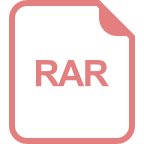









