img_all = np.stack(img_all)[:, :, :, ::-1].transpose(0, 3, 1, 2) # list to np.array and BGR to RGB
时间: 2024-06-07 08:10:18 浏览: 190
这段代码的作用是将一个由多个BGR图像构成的列表转换为一个RGB图像的numpy数组。具体来说,它首先使用`np.stack`将多个BGR图像叠加成一个四维的numpy数组,其中第一个维度表示图像的数量,第二和第三个维度表示图像的高度和宽度,第四个维度表示颜色通道(B、G、R)。然后,它使用`[:, :, :, ::-1]`将BGR通道的顺序反转为RGB通道的顺序。最后,它使用`transpose(0, 3, 1, 2)`将numpy数组的维度重新排列,以便它符合PyTorch期望的格式,即第一个维度表示批次大小,第二个维度表示颜色通道,第三个和第四个维度表示图像的高度和宽度。
相关问题
解释 img_all = np.stack(img_all)[:, :, :, ::-1].transpose(0, 3, 1, 2) # list to np.array and BGR to RGB
这段代码的作用是将一个包含多张图片的列表转换为一个四维的 numpy 数组,并将 BGR 通道顺序转换为 RGB 通道顺序。
具体来说,`np.stack(img_all)` 将列表中的图片堆叠起来,形成一个四维数组,其中第一维表示图片的数量,后三维表示图片的高度、宽度和通道数。
然后,`[:, :, :, ::-1]` 表示选择所有图片的所有行和列,并将通道维度的顺序反转,即将 BGR 通道顺序转换为 RGB 通道顺序。
最后,`transpose(0, 3, 1, 2)` 表示将四维数组的维度顺序转换为 `(数量, 通道数, 高度, 宽度)`,以符合常用的图片数据格式。这样处理后,就可以将这个 numpy 数组输入到模型中进行训练或推理了。
代码import os import numpy as np import nibabel as nib from PIL import Image # 创建保存路径 save_path = 'C:/Users/Administrator/Desktop/2D-LiTS2017' if not os.path.exists(save_path): os.makedirs(save_path) if not os.path.exists(os.path.join(save_path, 'image')): os.makedirs(os.path.join(save_path, 'image')) if not os.path.exists(os.path.join(save_path, 'label')): os.makedirs(os.path.join(save_path, 'label')) # 加载数据集 data_path = 'D:/BaiduNetdiskDownload/LiTS2017' img_path = os.path.join(data_path, 'Training Batch 1') label_path = os.path.join(data_path, 'Training Batch 2') # 转换图像 for file in sorted(os.listdir(img_path)): if file.endswith('.nii'): img_file = os.path.join(img_path, file) img = nib.load(img_file).get_fdata() img = np.transpose(img, (2, 0, 1)) # 转换为z, x, y for i in range(img.shape[0]): img_slice = img[i, :, :] img_slice = (img_slice - np.min(img_slice)) / (np.max(img_slice) - np.min(img_slice)) * 255 # 归一化到0-255 img_slice = img_slice.astype(np.uint8) img_slice = np.stack([img_slice]*3, axis=2) # 转换为三通道图像 img_name = file[:-4] + '' + str(i).zfill(3) + '.png' img_file_save = os.path.join(save_path, 'image', img_name) Image.fromarray(img_slice).save(img_file_save) # 转换标签 for file in sorted(os.listdir(label_path)): if file.endswith('.nii'): label_file = os.path.join(label_path, file) label = nib.load(label_file).get_fdata() label = np.transpose(label, (2, 0, 1)) # 转换为z, x, y for i in range(label.shape[0]): label_slice = label[i, :, :] label_slice[label_slice == 1] = 255 # 肝脏灰度值设为255 label_slice[label_slice == 2] = 128 # 肝脏肿瘤灰度值设为128 label_slice = label_slice.astype(np.uint8) label_name = file[:-4] + '' + str(i).zfill(3) + '.png' label_file_save = os.path.join(save_path, 'label', label_name) Image.fromarray(label_slice).save(label_file_save)出现scaled = scaled.astype(np.promote_types(scaled.dtype, dtype), copy=False) MemoryError错误,怎么修改?给出完整代码
这个错误通常意味着你的计算机没有足够的内存来处理数组。可能需要减少转换图像的尺寸或者使用更高效的算法。以下是一个可能的修改建议:
```python
import os
import numpy as np
import nibabel as nib
from PIL import Image
# 创建保存路径
save_path = 'C:/Users/Administrator/Desktop/2D-LiTS2017'
if not os.path.exists(save_path):
os.makedirs(save_path)
if not os.path.exists(os.path.join(save_path, 'image')):
os.makedirs(os.path.join(save_path, 'image'))
if not os.path.exists(os.path.join(save_path, 'label')):
os.makedirs(os.path.join(save_path, 'label'))
# 加载数据集
data_path = 'D:/BaiduNetdiskDownload/LiTS2017'
img_path = os.path.join(data_path, 'Training Batch 1')
label_path = os.path.join(data_path, 'Training Batch 2')
# 转换图像
for file in sorted(os.listdir(img_path)):
if file.endswith('.nii'):
img_file = os.path.join(img_path, file)
img = nib.load(img_file).get_fdata()
img = np.transpose(img, (2, 0, 1)) # 转换为z, x, y
for i in range(img.shape[0]):
img_slice = img[i, :, :]
img_slice = (img_slice - np.min(img_slice)) / (np.max(img_slice) - np.min(img_slice)) * 255 # 归一化到0-255
img_slice = img_slice.astype(np.uint8)
img_slice = np.stack([img_slice]*3, axis=2) # 转换为三通道图像
#减少图像大小
img_slice = np.array(Image.fromarray(img_slice).resize((512, 512), resample=Image.BICUBIC))
img_name = file[:-4] + '' + str(i).zfill(3) + '.png'
img_file_save = os.path.join(save_path, 'image', img_name)
Image.fromarray(img_slice).save(img_file_save)
# 转换标签
for file in sorted(os.listdir(label_path)):
if file.endswith('.nii'):
label_file = os.path.join(label_path, file)
label = nib.load(label_file).get_fdata()
label = np.transpose(label, (2, 0, 1)) # 转换为z, x, y
for i in range(label.shape[0]):
label_slice = label[i, :, :]
label_slice[label_slice == 1] = 255 # 肝脏灰度值设为255
label_slice[label_slice == 2] = 128 # 肝脏肿瘤灰度值设为128
label_slice = label_slice.astype(np.uint8)
label_name = file[:-4] + '' + str(i).zfill(3) + '.png'
label_file_save = os.path.join(save_path, 'label', label_name)
Image.fromarray(label_slice).save(label_file_save)
```
这里我们使用了 `PIL.Image` 中的 `resize()` 函数来减少图像大小。`resample=Image.BICUBIC` 用于指定插值方法,这里使用了 Bicubic 插值。这个方法可以保留图像的细节,并且可以减少内存使用。你也可以尝试使用其他插值方法。
阅读全文
相关推荐
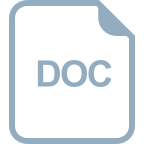
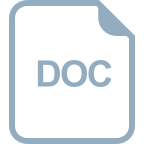
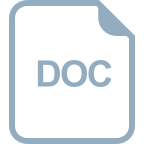

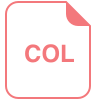
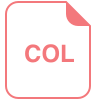
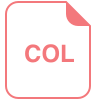
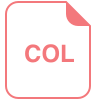
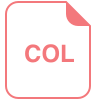
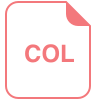






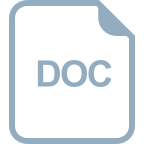