C#二维数组输入
时间: 2023-07-12 08:11:18 浏览: 75
可以使用以下代码实现C#二维数组的输入:
```csharp
int[,] array = new int[3, 3]; // 定义一个3行3列的二维数组
// 循环输入数组中的每个元素
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
Console.WriteLine("请输入第{0}行第{1}列的数据:", i + 1, j + 1);
array[i, j] = int.Parse(Console.ReadLine());
}
}
```
以上代码定义了一个3行3列的二维数组,然后通过嵌套的for循环,分别输入数组中每个元素的值。其中,`Console.ReadLine()`方法用于读取用户输入的数据,并将其转换为整型数据类型,以便存储到数组中。
相关问题
C#输入并输出二维数组
可以使用以下代码进行输入和输出二维数组:
```csharp
// 定义一个二维数组
int[,] arr = new int[2, 3];
// 输入二维数组的值
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 3; j++)
{
Console.Write("请输入[{0},{1}]的值:", i, j);
arr[i, j] = int.Parse(Console.ReadLine());
}
}
// 输出二维数组的值
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 3; j++)
{
Console.Write("{0}\t", arr[i, j]);
}
Console.WriteLine();
}
```
上述代码中,我们先定义了一个名为`arr`的二维数组,然后通过两个`for`循环进行输入和输出。在输入时,我们依次输入每个元素的值,并将其赋值给对应的数组元素;在输出时,我们同样通过两个`for`循环遍历整个数组,并通过`Console.Write`进行输出。注意,在输出每行后,我们还调用了`Console.WriteLine`进行换行,以保证输出结果的格式正确。
c# 字符串读入与读出二维数组
假设你有一个二维数组 `arr`,可以使用以下代码将其转换为字符串并输出:
```csharp
StringBuilder sb = new StringBuilder();
for (int i = 0; i < arr.GetLength(0); i++)
{
for (int j = 0; j < arr.GetLength(1); j++)
{
sb.Append(arr[i, j] + " ");
}
sb.AppendLine();
}
Console.WriteLine(sb.ToString());
```
这将在控制台中输出一个字符串,其中每行表示一个一维数组,每个数字用空格分隔。
要从字符串中读取并填充二维数组,可以使用以下代码:
```csharp
string input = "1 2 3\n4 5 6\n7 8 9\n"; // 假设这是输入字符串
string[] lines = input.Split(new[] { '\r', '\n' }, StringSplitOptions.RemoveEmptyEntries);
int[,] arr = new int[lines.Length, lines[0].Split(' ').Length];
for (int i = 0; i < lines.Length; i++)
{
string[] parts = lines[i].Split(' ');
for (int j = 0; j < parts.Length; j++)
{
arr[i, j] = int.Parse(parts[j]);
}
}
```
这将从输入字符串中解析出每个数字,并将它们逐个填充到二维数组中。
相关推荐
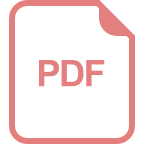












