编写一个程序,学生一起买小吃,共花钱50元,其中每个大学生花3元,每个中学生花2元,每个小学生花1元。现输入学生总人数30,问大、中、小学生的人数分配共有多少种不同的解(去掉某类学生数为0的解)?C语言解答
时间: 2024-11-07 20:24:15 浏览: 13
在C语言中,解决这个问题可以采用动态规划的方法。我们可以创建三个变量分别表示大学生、中学生和小学生的数量,然后遍历所有可能的组合,满足条件总花费为50元。因为每个类别的人数之和等于30,所以我们需要找到所有组合,使得3*大学生数量 + 2*中学生数量 + 1*小学生数量 = 50。
以下是C语言的一个简单实现:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_STUDENTS 30
bool isValid(int students[3], int total) {
return (students[0] * 3 + students[1] * 2 + students[2]) == total;
}
void countSolutions(int students[], int index, int total) {
if (index == 3) {
if (isValid(students, total)) {
printf("大学生: %d, 中学生: %d, 小学生: %d\n", students[0], students[1], students[2]);
// 记录并递增解决方案计数
solutions++;
}
return;
}
for (int i = 0; i <= total && i <= MAX_STUDENTS - students[index]; ++i) {
students[index] = i;
countSolutions(students, index + 1, total);
}
students[index] = 0; // 回溯到上一步
}
int main() {
int totalMoney = 50;
int students[MAX_STUDENTS];
int solutions = 0;
countSolutions(students, 0, totalMoney);
printf("不同的解共有 %d 种。\n", solutions);
return 0;
}
```
运行此程序会输出所有可能的大学生、中学生和小学生的人数组合,并计算出总的组合种类。注意,由于搜索空间较大,这个算法可能会生成大量的结果,实际应用中可能需要优化。
阅读全文
相关推荐
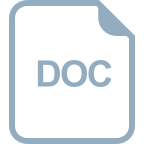
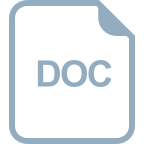
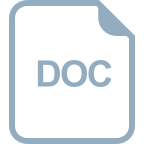



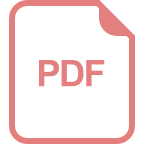
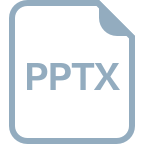
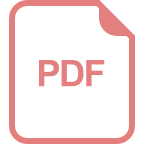
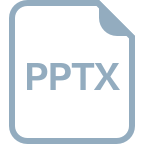
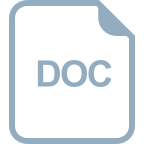
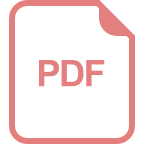
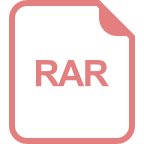
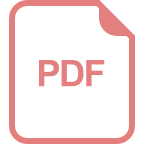
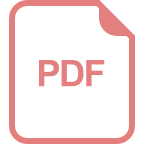
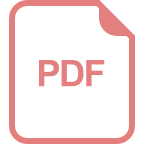
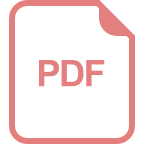