请统计在某个给定范围[m, n]的所有整数中,数字 n 出现的次数.
时间: 2024-05-02 10:17:14 浏览: 10
我们可以将这道题分成两个子问题:首先,我们需要找到在给定范围 [m, n] 中数字 n 出现的总次数;其次,我们需要将这个总次数拆分成各个位数上数字 n 出现的次数。
对于第一个子问题,我们可以遍历 [m, n] 中的每一个数字,计算其中数字 n 出现的次数,并累加到总次数中。
对于第二个子问题,我们可以将数字 n 拆分成各个位数上的数字,然后分别统计每个位数上数字 n 出现的次数。具体地,假设数字 n 有 k 位,我们可以使用一个数组 count 来记录在个位、十位、百位......上数字 n 出现的次数,然后将其累加起来即可。
下面是代码实现:
```python
def count_digit(n, d):
"""
统计数字 n 中数字 d 出现的次数
"""
count = 0
while n > 0:
if n % 10 == d:
count += 1
n //= 10
return count
def count_range(m, n, d):
"""
统计在范围 [m, n] 中数字 d 出现的次数
"""
count = 0
for i in range(m, n+1):
count += count_digit(i, d)
return count
def count_range_all_digits(m, n):
"""
统计在范围 [m, n] 中所有数字出现的次数
"""
count = [0] * 10 # 初始化 count 数组
for d in range(10):
count[d] = count_range(m, n, d)
return count
```
其中,`count_digit(n, d)` 函数用于统计数字 n 中数字 d 出现的次数;`count_range(m, n, d)` 函数用于统计在范围 [m, n] 中数字 d 出现的次数;`count_range_all_digits(m, n)` 函数用于统计在范围 [m, n] 中所有数字出现的次数,并返回一个包含 0~9 共 10 个数字在范围 [m, n] 中出现次数的列表 count。
相关推荐
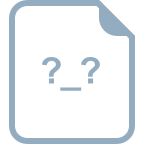











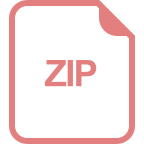
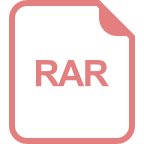