上面的代码只需要python和pygame就可以运行了吗
时间: 2024-02-05 19:03:29 浏览: 62
是的,上面的代码只需要安装好Python和Pygame库就可以运行了。
首先,确保你经安装了Python。你可以从Python官方网站(https://www.python.org/)下载并安装最新版本的Python。
然后,使用以下命令安装Pygame库:
```
pip install pygame
```
这将自动下载并安装Pygame库。
一旦你完成了这些步骤,就可以运行上面的代码了。在运行代码之前,确保你已经导入了`pygame`和`pygame.locals`模块。
如果一切顺利,你应该能够看到一个黑色的游戏窗口。这只是一个基础的游戏框架,你可以根据自己的需要进行修改和扩展。
希望这个解释对你有帮助!如果你还有其他问题,请随时提问。
相关问题
python五子棋pygame代码
五子棋是一个双人对战的游戏,玩家轮流在棋盘上放置自己的棋子,当一方的棋子在横、竖、斜线上连成五个时,该方获胜。下面是一个简单的Python五子棋游戏代码,使用了pygame库:
首先,确保已经安装了pygame库,如果没有安装,可以使用以下命令安装:
```bash
pip install pygame
```
接下来是五子棋游戏的代码:
```python
import pygame
import sys
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
size = width, height = 800, 800
screen = pygame.display.set_mode(size)
pygame.display.set_caption("五子棋")
# 设置棋盘大小和棋子半径
board_size = 15
cell_size = 50
stone_radius = 20
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
# 初始化棋盘
board = [[0 for _ in range(board_size)] for _ in range(board_size)]
# 加载字体
font = pygame.font.Font(None, 36)
def draw_board():
screen.fill(WHITE)
for i in range(board_size):
pygame.draw.line(screen, BLACK, (cell_size // 2 + i * cell_size, cell_size // 2), (cell_size // 2 + i * cell_size, height - cell_size // 2), 2)
pygame.draw.line(screen, BLACK, (cell_size // 2, cell_size // 2 + i * cell_size), (width - cell_size // 2, cell_size // 2 + i * cell_size), 2)
def draw_stone(x, y, color):
pygame.draw.circle(screen, color, (cell_size // 2 + x * cell_size, cell_size // 2 + y * cell_size), stone_radius)
def check_win(x, y, color):
directions = [(-1, 0), (0, -1), (-1, -1), (-1, 1)]
for dx, dy in directions:
count = 1
for i in range(1, 5):
nx, ny = x + i * dx, y + i * dy
if 0 <= nx < board_size and 0 <= ny < board_size and board[nx][ny] == color:
count += 1
else:
break
for i in range(1, 5):
nx, ny = x - i * dx, y - i * dy
if 0 <= nx < board_size and 0 <= ny < board_size and board[nx][ny] == color:
count += 1
else:
break
if count >= 5:
return True
return False
def main():
global board
turn = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
i, j = x // cell_size, y // cell_size
if board[i][j] == 0:
board[i][j] = turn % 2
turn += 1
draw_stone(i, j, BLACK if turn % 2 == 0 else RED)
if check_win(i, j, BLACK if turn % 2 == 0 else RED):
print("Player", turn % 2 + 1, "wins!")
sys.exit()
draw_board()
draw_board()
pygame.display.flip()
pygame.time.delay(1000 // 60)
if __name__ == "__main__":
main()
```
运行这段代码,即可开始五子棋游戏。游戏中
python使用pygame有趣的代码
使用pygame编写游戏代码是非常有趣和刺激的。以下是一个简单的示例代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 创建游戏窗口
window_width = 800
window_height = 600
window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("打砖块")
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义游戏变量
paddle_width = 100
paddle_height = 10
paddle_x = window_width / 2 - paddle_width / 2
paddle_y = window_height - paddle_height - 20
paddle_speed = 5
ball_radius = 10
ball_x = window_width / 2
ball_y = window_height / 2
ball_speed_x = random.randint(-5, 5)
ball_speed_y = 5
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 移动挡板
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
paddle_x -= paddle_speed
if keys[pygame.K_RIGHT]:
paddle_x += paddle_speed
# 移动小球
ball_x += ball_speed_x
ball_y += ball_speed_y
# 碰撞检测
if ball_x < 0 or ball_x > window_width - ball_radius:
ball_speed_x = -ball_speed_x
if ball_y < 0 or ball_y > window_height - ball_radius:
ball_speed_y = -ball_speed_y
if paddle_x < ball_x < paddle_x + paddle_width and ball_y + ball_radius > paddle_y:
ball_speed_y = -ball_speed_y
# 清空窗口
window.fill(BLACK)
# 绘制挡板和小球
pygame.draw.rect(window, WHITE, (paddle_x, paddle_y, paddle_width, paddle_height))
pygame.draw.circle(window, RED, (ball_x, ball_y), ball_radius)
# 更新窗口显示
pygame.display.flip()
# 退出游戏
pygame.quit()
```
这是一个简单的打砖块游戏,玩家可以控制挡板在屏幕底部移动,通过击打小球使其反弹并消除砖块。代码中实现了小球和挡板的移动,以及碰撞检测和反弹效果。游戏运行时,用户可以按左右键控制挡板的移动,小球与窗口边界和挡板的碰撞会引发反弹效果。通过这个有趣的代码示例,你可以进一步研究pygame并编写更加复杂和有趣的游戏。
阅读全文
相关推荐
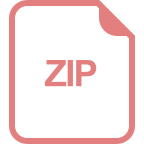
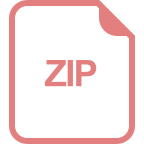
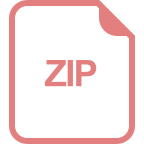













