python和pygame的A *
时间: 2023-07-20 16:30:33 浏览: 53
A*算法是一种寻路算法,可以用于游戏中的NPC寻找最短路径。在Python中,可以使用pygame库来实现A*算法。
下面是一个简单的A*算法实现的代码示例:
```python
import pygame
import heapq
# 地图宽度和高度
MAP_WIDTH = 800
MAP_HEIGHT = 600
# 格子宽度和高度
CELL_WIDTH = 20
CELL_HEIGHT = 20
# 地图中障碍物的位置,0表示空地,1表示障碍物
MAP = [
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 1, 1, 1, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
]
# 定义节点类
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.g = 0
self.h = 0
self.parent = None
def __lt__(self, other):
return self.g + self.h < other.g + other.h
def __eq__(self, other):
return self.x == other.x and self.y == other.y
# 计算节点到终点的曼哈顿距离
def manhattan_distance(node, end_node):
return abs(node.x - end_node.x) + abs(node.y - end_node.y)
# 获取节点的邻居节点
def get_neighbors(node):
neighbors = []
for dx in [-1, 0, 1]:
for dy in [-1, 0, 1]:
if dx == 0 and dy == 0:
continue
x = node.x + dx
y = node.y + dy
if x < 0 or x >= MAP_WIDTH or y < 0 or y >= MAP_HEIGHT:
continue
if MAP[x][y] == 1:
continue
neighbors.append(Node(x, y))
return neighbors
# A*算法
def a_star(start_node, end_node):
open_list = []
closed_list = set()
heapq.heappush(open_list, start_node)
while len(open_list) > 0:
current_node = heapq.heappop(open_list)
if current_node == end_node:
path = []
while current_node is not None:
path.append((current_node.x, current_node.y))
current_node = current_node.parent
return path[::-1]
closed_list.add(current_node)
for neighbor in get_neighbors(current_node):
if neighbor in closed_list:
continue
new_g = current_node.g + 1
if neighbor not in open_list or new_g < neighbor.g:
neighbor.g = new_g
neighbor.h = manhattan_distance(neighbor, end_node)
neighbor.parent = current_node
if neighbor not in open_list:
heapq.heappush(open_list, neighbor)
return None
# 主程序
def main():
pygame.init()
# 创建地图窗口
screen = pygame.display.set_mode((MAP_WIDTH, MAP_HEIGHT))
# 绘制地图
for x in range(0, MAP_WIDTH, CELL_WIDTH):
for y in range(0, MAP_HEIGHT, CELL_HEIGHT):
rect = pygame.Rect(x, y, CELL_WIDTH, CELL_HEIGHT)
if MAP[x // CELL_WIDTH][y // CELL_HEIGHT] == 1:
pygame.draw.rect(screen, (255, 0, 0), rect)
else:
pygame.draw.rect(screen, (255, 255, 255), rect)
# 设置起点和终点
start_node = Node(0, 0)
end_node = Node(9, 9)
# 运行A*算法
path = a_star(start_node, end_node)
# 绘制路径
for x, y in path:
rect = pygame.Rect(x * CELL_WIDTH, y * CELL_HEIGHT, CELL_WIDTH, CELL_HEIGHT)
pygame.draw.rect(screen, (0, 255, 0), rect)
# 刷新窗口
pygame.display.flip()
# 等待退出
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
return
if __name__ == '__main__':
main()
```
这段代码实现了一个简单的A*算法寻路,可以在pygame窗口中显示出地图和寻路路径。在运行代码之前,需要先安装pygame库。
相关推荐
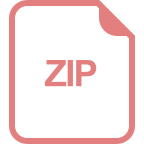














