torch.concatenate
时间: 2023-10-30 11:06:18 浏览: 147
torch.concatenate 是 PyTorch 中用于拼接张量的函数。它可以将多个张量沿指定的维度进行拼接。你可以传入一个包含多个张量的列表,并指定拼接的维度。例如:
```python
import torch
# 创建两个张量
x1 = torch.tensor([[1, 2, 3],
[4, 5, 6]])
x2 = torch.tensor([[7, 8, 9],
[10, 11, 12]])
# 沿着第 0 维度拼接
result = torch.cat([x1, x2], dim=0)
print(result)
# 输出:
# tensor([[ 1, 2, 3],
# [ 4, 5, 6],
# [ 7, 8, 9],
# [10, 11, 12]])
# 沿着第 1 维度拼接
result = torch.cat([x1, x2], dim=1)
print(result)
# 输出:
# tensor([[ 1, 2, 3, 7, 8, 9],
# [ 4, 5, 6, 10, 11, 12]])
```
这样,你就可以使用 torch.concatenate 来拼接多个张量。希望这个回答对你有帮助!如果你还有其他问题,请随时提问。
相关问题
torch.concatenate和torch.concat
在PyTorch中,`torch.cat`函数可以用来沿着指定的维度拼接张量。`torch.cat`的语法是:
```python
torch.cat(tensors, dim=0, out=None) -> Tensor
```
其中,`tensors`是要拼接的张量序列,`dim`是沿着哪个维度进行拼接,默认为0,表示在第0维度上拼接。`out`是输出张量,如果提供了输出张量,则会将结果拷贝到输出张量中。
`torch.cat`函数将拼接张量的所有维度都看作一个整体,因此它要求所有输入张量除了指定的拼接维度以外,其它维度都应该一样。如果输入张量的维度不一致,则可以使用`torch.stack`函数将它们堆叠成同样维度的张量后再拼接。
与`torch.cat`类似的函数还有`torch.stack`和`torch.chunk`。`torch.stack`函数将输入张量序列沿着新的维度堆叠起来,而`torch.chunk`函数将张量沿着指定维度分成若干块。
torch.cat和torch.concatenate
torch.cat和torch.concatenate是PyTorch中用于连接张量的函数。它们的功能类似,都可以用于沿指定维度连接多个张量。但是,它们有一些细微的差别。
torch.cat接受一个张量列表作为输入,并在指定维度上拼接它们。例如,如果有两个形状为(3, 4)的张量A和B,你可以使用torch.cat([A, B], dim=0)将它们在第0维上连接起来,得到一个形状为(6, 4)的张量。
torch.concatenate也是用于连接张量,但它接受的输入是一个元组或列表,并且可以指定连接的维度。与torch.cat不同的是,torch.concatenate不会自动扩展输入张量的维度。因此,如果输入张量的维度不匹配,torch.concatenate将引发错误。
总结来说,torch.cat更常用且灵活,而torch.concatenate则更严格要求输入张量的维度匹配。
阅读全文
相关推荐
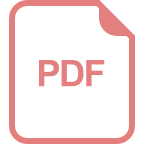
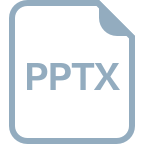
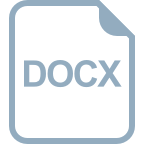














