中缀表达式计算c语言
时间: 2023-08-25 09:12:49 浏览: 104
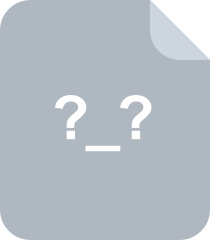
c语言中缀表达式计算
中缀表达式计算可以通过将中缀表达式转换为后缀表达式,然后再利用栈来计算后缀表达式的值。具体的实现步骤如下:
1. 定义一个运算符栈和一个操作数栈;
2. 从左向右扫描中缀表达式,如果是数字,则直接入操作数栈,如果是运算符,则按照下列规则处理:
a. 如果运算符栈为空,或者栈顶运算符为左括号,则直接入栈;
b. 如果优先级比栈顶运算符的优先级高,则直接入栈;
c. 如果优先级比栈顶运算符的优先级低或者相等,则将栈顶运算符弹出,并将其加入到后缀表达式中,然后重复步骤2,直到满足条件a或b为止;
d. 如果是左括号,则直接入栈;
e. 如果是右括号,则将运算符栈中的运算符弹出并加入到后缀表达式中,直到弹出左括号为止;
3. 如果扫描完毕,但是运算符栈中还有运算符,则依次弹出并加入到后缀表达式中;
4. 利用操作数栈和后缀表达式来计算表达式的值。
以下是一个示例代码,可以实现中缀表达式计算:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_SIZE 100
typedef struct {
int top;
char data[MAX_SIZE];
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_SIZE - 1;
}
int push(Stack *s, char ch) {
if (is_full(s)) {
return 0;
}
s->data[++s->top] = ch;
return 1;
}
char pop(Stack *s) {
if (is_empty(s)) {
return '\0';
}
return s->data[s->top--];
}
char peek(Stack *s) {
if (is_empty(s)) {
return '\0';
}
return s->data[s->top];
}
int is_operator(char ch) {
return ch == '+' || ch == '-' || ch == '*' || ch == '/';
}
int get_priority(char ch) {
if (ch == '+' || ch == '-') {
return 1;
} else if (ch == '*' || ch == '/') {
return 2;
}
return 0;
}
int infix_to_postfix(char *infix, char *postfix) {
Stack op_stack;
init(&op_stack);
int i, j;
for (i = 0, j = 0; infix[i]; i++) {
if (isdigit(infix[i])) {
postfix[j++] = infix[i];
} else if (is_operator(infix[i])) {
while (!is_empty(&op_stack) && peek(&op_stack) != '('
&& get_priority(peek(&op_stack)) >= get_priority(infix[i])) {
postfix[j++] = pop(&op_stack);
}
push(&op_stack, infix[i]);
} else if (infix[i] == '(') {
push(&op_stack, infix[i]);
} else if (infix[i] == ')') {
while (!is_empty(&op_stack) && peek(&op_stack) != '(') {
postfix[j++] = pop(&op_stack);
}
if (!is_empty(&op_stack) && peek(&op_stack) == '(') {
pop(&op_stack);
}
}
}
while (!is_empty(&op_stack)) {
postfix[j++] = pop(&op_stack);
}
postfix[j] = '\0';
return 1;
}
int calculate(char *postfix) {
Stack num_stack;
init(&num_stack);
int i, num;
for (i = 0; postfix[i]; i++) {
if (isdigit(postfix[i])) {
num = postfix[i] - '0';
push(&num_stack, num);
} else if (is_operator(postfix[i])) {
int num2 = pop(&num_stack);
int num1 = pop(&num_stack);
switch (postfix[i]) {
case '+':
push(&num_stack, num1 + num2);
break;
case '-':
push(&num_stack, num1 - num2);
break;
case '*':
push(&num_stack, num1 * num2);
break;
case '/':
push(&num_stack, num1 / num2);
break;
}
}
}
return pop(&num_stack);
}
int main() {
char infix[MAX_SIZE], postfix[MAX_SIZE];
printf("Enter an infix expression: ");
scanf("%s", infix);
infix_to_postfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
printf("Result: %d\n", calculate(postfix));
return 0;
}
```
注意:这段代码中没有处理错误情况,例如表达式不合法等情况,实际使用中需要根据具体情况进行处理。
阅读全文
相关推荐

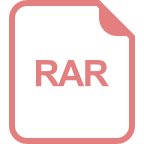
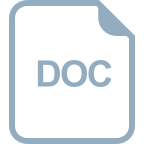






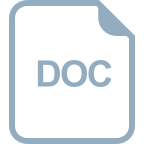
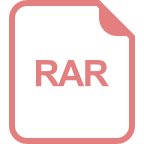
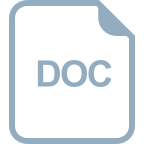
