springboot调用第三方接口用什么技术
时间: 2024-05-24 12:10:19 浏览: 123
SpringBoot可以使用多种技术来调用第三方接口,以下是一些常见的技术:
1. RestTemplate:RestTemplate是Spring提供的一个HTTP客户端,可以用于发送HTTP请求并接收响应。使用RestTemplate可以轻松地调用RESTful风格的接口。
2. Feign:Feign是一个声明式的HTTP客户端,使得编写Web服务客户端变得更加简单。它集成了Ribbon和Hystrix,可以实现负载均衡和服务降级。
3. WebClient:WebClient是Spring 5引入的新的反应式Web客户端,可以用于异步调用第三方接口,支持Reactive Streams API。
4. Retrofit:Retrofit是一个针对RESTful API的类型安全的HTTP客户端,可以使用注解来定义HTTP请求参数和返回值,可以将Java接口转换为HTTP API。
5. Apache HttpClient:Apache HttpClient是一个基于Java的HTTP客户端库,可以用于发送HTTP请求并接收响应。它提供了多种配置选项和可扩展性。
相关问题
springboot 调用第三方接口
Spring Boot可以通过RestTemplate或FeignClient来调用第三方接口。
1. RestTemplate
RestTemplate是Spring提供的一个HTTP客户端工具,可以用来发送HTTP请求并接收响应。使用RestTemplate调用第三方接口的步骤如下:
1)在Spring Boot项目中添加RestTemplate依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
2)在代码中创建RestTemplate对象,并使用它发送HTTP请求:
```java
RestTemplate restTemplate = new RestTemplate();
String result = restTemplate.getForObject("http://api.example.com/user/1", String.class);
```
2. FeignClient
FeignClient是Spring Cloud提供的一个HTTP客户端工具,可以用来发送HTTP请求并接收响应。使用FeignClient调用第三方接口的步骤如下:
1)在Spring Boot项目中添加FeignClient依赖:
```xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
```
2)在代码中创建FeignClient接口,并使用它发送HTTP请求:
```java
@FeignClient(name = "example", url = "http://api.example.com")
public interface ExampleClient {
@GetMapping("/user/{id}")
String getUser(@PathVariable("id") Long id);
}
ExampleClient exampleClient = Feign.builder()
.encoder(new JacksonEncoder())
.decoder(new StringDecoder())
.target(ExampleClient.class, "http://api.example.com");
String result = exampleClient.getUser(1L);
```
以上是两种常用的调用第三方接口的方式,具体使用哪种方式取决于具体的业务需求和技术栈。
springboot调用第三方接口
Spring Boot是一个高效的开发框架,它能够轻松地调用第三方接口。下面是使用Spring Boot调用第三方接口的步骤:
1. 导入相关依赖
在pom.xml文件中添加相关依赖,例如:
```
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>3.14.6</version>
</dependency>
</dependencies>
```
其中,spring-boot-starter-web是Spring Boot的web启动器,okhttp是一个HTTP客户端库,可以用来发送HTTP请求。
2. 编写调用代码
在Java代码中使用OkHttp发送HTTP请求,例如:
```
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
// ...
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://api.example.com/user")
.build();
Response response = client.newCall(request).execute();
String responseBody = response.body().string(); // 获取响应体
```
在这个示例中,我们使用OkHttpClient创建一个HTTP客户端,并使用Request构建器创建一个HTTP请求对象。然后,我们使用HTTP客户端发送请求,并获取响应体。在实际使用中,您需要根据具体的接口文档和业务需求来编写代码。
3. 配置接口地址和参数
在实际使用中,您需要根据具体的接口文档和业务需求来配置接口地址和参数。您可以使用properties或yaml文件配置接口地址和参数,例如:
```
api.url=https://api.example.com/user
api.key=your_api_key
```
然后,在Java代码中使用@Value注解获取配置值,例如:
```
import org.springframework.beans.factory.annotation.Value;
// ...
@Value("${api.url}")
private String apiUrl;
@Value("${api.key}")
private String apiKey;
// ...
OkHttpClient client = new OkHttpClient();
HttpUrl.Builder urlBuilder = HttpUrl.parse(apiUrl).newBuilder();
urlBuilder.addQueryParameter("key", apiKey);
String url = urlBuilder.build().toString();
Request request = new Request.Builder()
.url(url)
.build();
Response response = client.newCall(request).execute();
String responseBody = response.body().string();
```
在这个示例中,我们使用@Value注解获取配置值,并使用HttpUrl构建器构建URL,并添加查询参数。然后,我们使用HTTP客户端发送请求,并获取响应体。
4. 处理响应
在实际使用中,您需要根据具体的接口文档和业务需求来处理响应。您可以使用Jackson或Gson等JSON处理库来解析响应数据,例如:
```
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.JsonNode;
// ...
ObjectMapper objectMapper = new ObjectMapper();
JsonNode responseJson = objectMapper.readTree(responseBody);
String name = responseJson.get("name").asText(); // 获取name字段的值
```
在这个示例中,我们使用ObjectMapper解析JSON响应体,并获取响应中的name字段的值。
以上就是使用Spring Boot调用第三方接口的步骤。为了保证代码的可靠性和可维护性,建议您在编写代码之前,先仔细阅读接口文档,并进行必要的测试和调试。
阅读全文
相关推荐
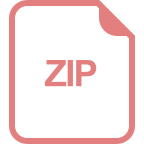
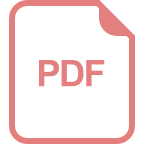






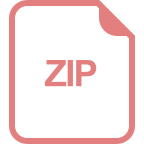






