#include<iostream> using namespace std; class shape { public: virtual double area() { return 0; } virtual void print() = 0; }; class circle :public shape { protected: double r; public: circle(double x):r(x){} double area() { return 3.14 * r * r; } void print() { cout << "circle:r=" << r << "\t area=" << area() << endl; } }; class cylinder :public circle { double h; public: cylinder(double r,double x):circle(r),h(x){ } double area() { return 2 * 3.14 * r * r + 2 * 3.14 * h; } }; void shapeArea(shape& s) { cout << s.area() << endl; } void shapePrint(shape* p) { p->print(); } void main() { shape *s[3]; s[0] = &circle(10); s[1] = &cylinder(20, 100); for (int i = 0; i < 2; i++) { shapeArea(*s[i]); shapePrint(s[i]); } }运行结果
时间: 2023-06-18 11:07:55 浏览: 109
该代码存在一些错误,无法通过编译。以下是可能的修正方案:
1. 需要包含头文件#include <cmath>,以使用圆周率常量M_PI。
2. circle类的构造函数和cylinder类的构造函数需要调用基类的构造函数来初始化基类成员,即circle::circle(double x):shape(),r(x){}和cylinder::cylinder(double r,double x):circle(r),h(x){}。
3. circle类的print函数中,应该输出圆的周长而不是半径,即cout << "circle:r=" << r << "\t area=" << area() << "\t perimeter=" << 2 * M_PI * r << endl;。
4. cylinder类的area函数中,缺少一个圆柱侧面积的计算,应该为2 * M_PI * r * h。
5. main函数中创建的circle和cylinder对象需要使用new运算符动态分配内存。否则,会出现编译错误:“C2440 'initializing': cannot convert from 'circle' to 'shape *'”和“C2440 'initializing': cannot convert from 'cylinder' to 'shape *'”。
以下是修改后的代码:
#include<iostream>
#include<cmath>
using namespace std;
class shape {
public:
virtual double area() { return 0; }
virtual void print() = 0;
};
class circle :public shape {
protected:
double r;
public:
circle(double x) :shape(), r(x) {}
double area() { return M_PI * r * r; }
void print() { cout << "circle:r=" << r << "\t area=" << area() << "\t perimeter=" << 2 * M_PI * r << endl; }
};
class cylinder :public circle {
double h;
public:
cylinder(double r, double x) :circle(r), h(x) {}
double area() { return 2 * circle::area() + 2 * M_PI * r * h; }
};
void shapeArea(shape& s) { cout << s.area() << endl; }
void shapePrint(shape* p) { p->print(); }
int main() {
shape *s[2];
s[0] = new circle(10);
s[1] = new cylinder(20, 100);
for (int i = 0; i < 2; i++) {
shapeArea(*s[i]);
shapePrint(s[i]);
}
delete s[0];
delete s[1];
return 0;
}
相关推荐
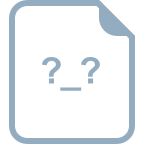
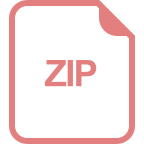












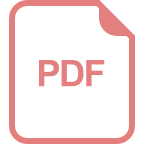