第五题: 以下为定义的图形继承体系: class Shape{ public: // . . . virtual double area(){} }; class Circle:public Shape{ public: double area(){…} // . . . }; class Triangle:public Shape{ public: double area(){…} // . . . }; // . . . 以下为容器类: class Manage{ shape *a[100]; public: // . . . } 要求完成Manage类的拷贝构造函数,实现深度拷贝;可以改动所有的类,并给出main函数测试程序。
时间: 2023-06-23 09:10:14 浏览: 47
为了实现深度拷贝,需要对Manage类的拷贝构造函数进行重载,具体实现如下:
```c++
#include <iostream>
using namespace std;
class Shape{
public:
virtual double area(){}
};
class Circle: public Shape{
public:
double area(){ return 0.0; }
};
class Triangle: public Shape{
public:
double area(){ return 0.0; }
};
class Manage{
Shape *a[100];
int size;
public:
// 默认构造函数
Manage(): size(0){}
// 拷贝构造函数
Manage(const Manage &other){
size = other.size;
for(int i = 0; i < size; i++){
if(dynamic_cast<Circle*>(other.a[i])){
a[i] = new Circle(*(Circle*)other.a[i]);
}
else{
a[i] = new Triangle(*(Triangle*)other.a[i]);
}
}
}
// 析构函数
~Manage(){
for(int i = 0; i < size; i++){
delete a[i];
}
}
// 添加图形
void add(Shape *s){
a[size++] = s;
}
// 打印所有图形的面积
void print(){
for(int i = 0; i < size; i++){
cout << "Area of shape " << i+1 << ": " << a[i]->area() << endl;
}
}
};
int main(){
Manage m1;
m1.add(new Circle());
m1.add(new Triangle());
m1.print();
Manage m2 = m1; // 调用拷贝构造函数
m2.print();
return 0;
}
```
在拷贝构造函数中,对于每个元素进行判断,如果是Circle类型,则调用Circle的拷贝构造函数进行深度拷贝,否则调用Triangle的拷贝构造函数。同时,在析构函数中,需要对所有的Shape指针进行释放。最后,在main函数中,我们定义了两个Manage对象m1和m2,将m1赋值给m2,测试其拷贝构造函数是否实现了深度拷贝。
相关推荐
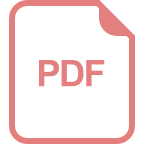
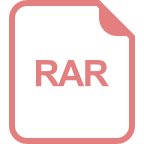















